Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial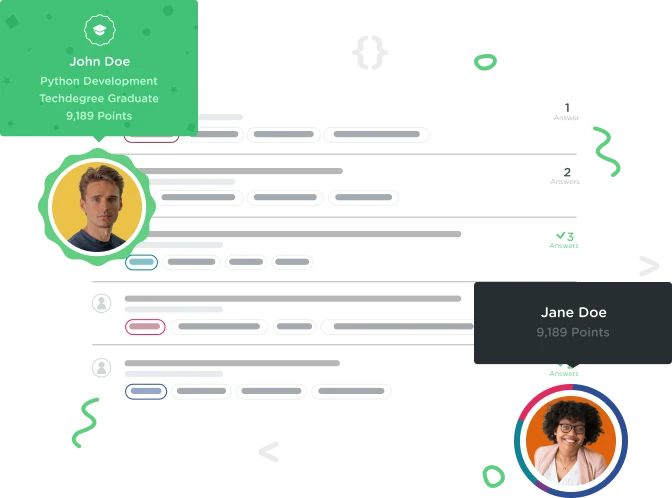
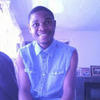
Bernard Chiyangwa
7,094 PointsChallenge Task 2 of 2 Inside the stringGPA() method, convert the value of the gpa string and return it.
Challenge Task 2 of 2
Inside the stringGPA() method, convert the value of the gpa property to a string and return it.
class Student {
constructor(gpa,stringGPA){
this.gpa = gpa;
this.stringGPA = stringGPA;
}
stringGPA() {}
};
const student = new Student(3.9);
this.Student returnValue("3.9");
14 Answers
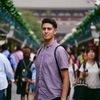
Julian Cobos
10,646 PointsQuestion: Inside the stringGPA() method, convert the value of the gpa property to a string and return it.
Your goal is to grab the gpa which is currently a number and turn it into a string. example = 12345 to "12345".
Answer:
class Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA() {
return String(this.gpa);
}
}
const student = new Student(3.9);
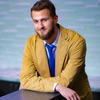
Alex Franklin
12,403 PointsAt what point in ANY video in the lessons up to this challenge reference the use of, "toString()" ? ? ?
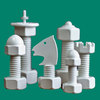
Steven Parker
231,269 PointsWhile it works, it's not necessary to use "toString()" to solve the challenge.

Jmaisin Williams
8,263 PointsExactly!!!

Riaz Khan
5,320 Pointsclass Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA(){
return this.gpa.toString();
}
}
const student = new Student(3.9);
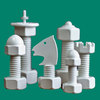
Steven Parker
231,269 Points Explicit answers without any explanation are strongly discouraged by Treehouse and may be subject to redaction by staff or moderators.

Darren Ward
12,025 PointsComing off of the front end web dev track, it feels like not everything has been explained as well as in the other tracks by the other tutors.
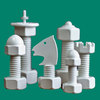
Steven Parker
231,269 PointsIt looks like you got a bit off-track The instructions say "Inside the stringGPA() method", so all the code you add for this task should all go between the braces you added in task 1.
They also say, "convert the value of the gpa property to a string and return it.". So you'll need to reference the property already created in the constructor, convert it to a string, and "return" it. You won't need to create a new property (and a property cannot have the same name as a method).
Give it another try with these things in mind.
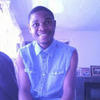
Bernard Chiyangwa
7,094 Pointsi dont seem to understand ive been doing this challenge for a while now
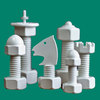
Steven Parker
231,269 PointsIn task 1, you created a new method:
stringGPA() {}
your method includes this pair of braces
So far task 2, all new code needs to go in between those braces. So the line you added to the constructor, and the line you added at the end should not be there.
Then, the code you add won't have any literal values in it. You need to refer to the existing property named "gpa".
You might want to re-watch the last video and take another look at the example shown there.
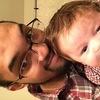
John Oconnell
13,807 PointsThis should be the top answer for sure, thanks for this. Explains WHY the code does what it does.
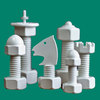
Steven Parker
231,269 PointsThanks, I always try to explain but let the student still solve it for themselves. I think it creates a better learning experience.
But as you see, some students still prefer explicit answers. ¯\_(ツ)_/¯
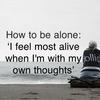
learning67learning67
6,479 PointsstringGPA() {
return this.gpa.toString();
}
Add this code to stringGPA method.
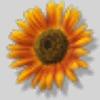
Noelle Lewis
7,895 PointsDid anyone figure this out? Because Im having a hard time
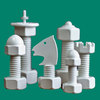
Steven Parker
231,269 PointsSeveral answers shown above have viable solutions!
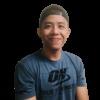
Faiz Hamdan
5,215 PointsstringGPA () {
return String(this.gpa) // This will spit out ' this is not a function '
}
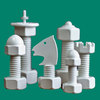
Steven Parker
231,269 PointsIt would by itself. That definition style can only be used as part of a class definition (as in Julian's example).
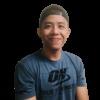
Faiz Hamdan
5,215 PointsstringGPA () {
return this.gpa.toString() // Also spit out " student.stringGPA is not a function "
}
I've try my very best. After researching from various forums, everything i do spit out This is not a function
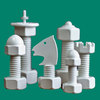
Steven Parker
231,269 PointsI tried pasting that directly into the challenge and it passed. Is it possible you've accidentally changed something else in the provided code?
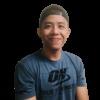
Faiz Hamdan
5,215 PointsO gawd! School boy mistake! I used a visual studio code to write my code rather than using the given workspace TreeHouse provide. And i copied everything that was there and paste it. Thanks again for helping out Steve!
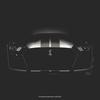
Sundar Maharjan
6,740 Pointsclass Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA() {
return String(this.gpa);
}
}
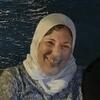
Salwa Elmohandes
Full Stack JavaScript Techdegree Graduate 20,240 Pointsreturn this.gpa=‘gpa’;

Stephanie Czetli
13,180 PointsMy answer is similar to Salwa Elmohandes', and while her answer worked for her, it didn't work for me. By changing the single quotes ' ' around gpa to double quotes " ", it worked!
stringGPA() { return this.gpa = "gpa"; }

Jennifer Sanchez
8,536 PointsI used a template literal and it worked!
class Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA() {
return (${this.gpa}
);
}
}
const student = new Student(3.9);
Mauricio Hernandez
7,208 PointsMauricio Hernandez
7,208 PointsThank you, God bless you and your family.
God bless everyone and your family.