Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial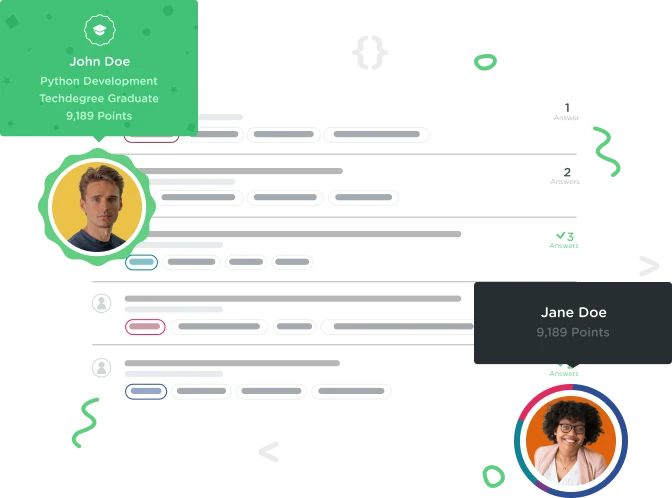

Michael Leonard
1,279 PointsChallenge Task 2 of 3 Question
Question asks: Use .remove() and/or del to remove the string, boolean, and list members of the_list.
This seems to work for me in the terminal. Is this wrong?
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
crap = the_list.pop(3)
the_list.insert(0, crap)
print(the_list)
length = len(the_list)
for i in range(0, length):
i = 0
del the_list[i]
i += 1
print(the_list)
3 Answers

Raghu Savalagi
1,511 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
the_list.insert(0, the_list.pop(3)) print the_list
for i in range(0, len(the_list)): try: del the_list[0] except IndexError: pass print the_list

Chris Bennett
7,499 PointsThis is my solution to the problem.
Another way is to use the reverse() function on the list and iterate through it backwards.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
temp_list = []
for item in the_list:
if type(item) == int:
temp_list.append(item)
the_list = temp_list
print the_list

Michael Leonard
1,279 PointsThanks for the help!
Maybe I am misunderstanding the question. I interpreted it as "Delete all items in the list". Is this incorrect? What is "and list members of the_list" referring to?
Thanks!

Chris Bennett
7,499 PointsIt is asking you to basically delete everything that isn't an int from the list (the boolean, string and list objects).
At the end of "the_list" (list[5]) is a list, that needs to be deleted. The wording is a bit confusing.
You can see what types things are by using the type() function. type(the_list[5]) would return list.