Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial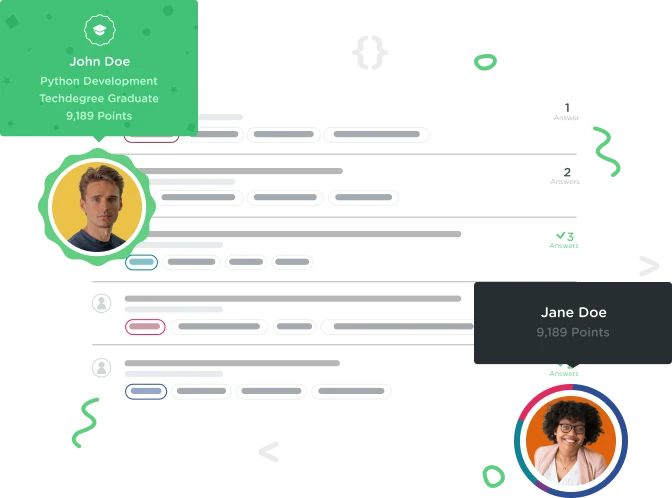

Timothy Bass
888 PointsChallenge Task 2 of 3 - works in workspace.... but sadly
So this works in the work space with an output of [1, 2, 3, [1, 2, 3]]
But not in the 'homework' part of it.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, (the_list.pop(3)))
for item in the_list:
if isinstance(item, str) or isinstance(item, bool):
the_list.remove(item)
print(the_list)
1 Answer
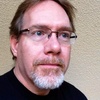
Chris Freeman
Treehouse Moderator 68,423 PointsKudos for taking taken a unique approach. Two aspects need fixing.
- Also check for the type
list
- and it's almost never a good idea to manipulate the list you looping over as it causes index errors Use a copy instead:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, (the_list.pop(3)))
for item in the_list[:]: # <-- use [:] to signify a copy
if isinstance(item, str) or isinstance(item, bool) or isinstance(item, list): # <-- added 'list' check
the_list.remove(item)
Also, the print(the_list)
is unnecessary.
Others that have solved this challenged used a more brute force approach of:
the_list.remove(False)
the_list.remove("a")
the_list.remove([1, 2, 3])
dmitryburyi
225 Pointsdmitryburyi
225 PointsChris, could you please briefly explain why the code below doesn't remove the list ([1,2,3]) in case if a copy ([:]) is not created. Thanks.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsExpanding the code to add print statements and clarify the test case:
Results in:
Removing copy:
Results in:
The list
[1, 2, 3]
is not removed because the container iterated over by thefor
loop is shrinking as each item is removed.In the loop, the next item from the iterable
the_list
is referenced by index: 0, 1, 2, 3, ....Using
[1, "a", 2, 3, 4, False, [1, 2, 3]]
[1, 2, 3]
shifts to index [4]The item
[1, 2, 3]
had shifted to index [4] due to the items removed effectively being skipped in the loop. Checking2
was also skipped.dmitryburyi
225 Pointsdmitryburyi
225 PointsThanks, Chris!