Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial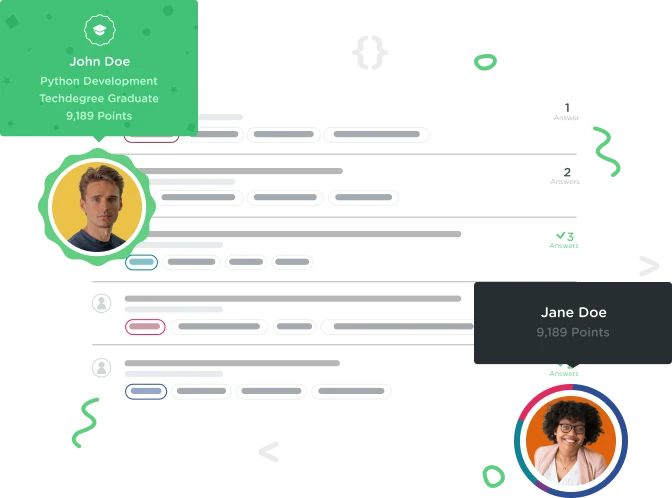

Harrison Cassedy
Java Web Development Techdegree Graduate 12,031 PointsChallenge Task 3 of 3 Implement the save method in ContactServiceImpl by calling the DAO's respective method.
Not sure where to go from here.
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactDao {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
package com.teamtreehouse.contactmgr.dao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class ContactDaoImpl implements ContactDao {
@Autowired
private SessionFactory sessionFactory;
@Override
public List<Contact> findAll() {
Session session = sessionFactory.openSession();
List<Contact> contacts = session.createCriteria(Contact.class).list();
session.close();
return contacts;
}
@Override
public void save(Contact contact) {
Session session = sessionFactory.openSession ();
session.beginTransaction();
session.save(category);
session.getTransaction().commit();
session.close();
}
@Override
public Contact findById(Long id) {
Session session = sessionFactory.openSession();
Contact c = session.get(Contact.class, id);
session.close();
return c;
}
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.model.Contact;
import java.util.List;
public interface ContactService {
List<Contact> findAll();
Contact findById(Long id);
void save(Contact contact);
}
package com.teamtreehouse.contactmgr.service;
import com.teamtreehouse.contactmgr.dao.ContactDao;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ContactServiceImpl implements ContactService {
@Autowired
private ContactDao contactDao;
@Override
public List<Contact> findAll() {
return contactDao.findAll();
}
@Override
public void save(Category category) {
}
@Override
public Contact findById(Long id) {
return contactDao.findById(id);
}
}
1 Answer

Boban Talevski
24,793 PointsHi Harrison,
First of all, your implementation of the save method in ContactDaoImpl.java is wrong, not sure if you passed task 2 with it. When calling session's save method, you should use the contact parameter passed in instead of a category parameter which doesn't exist in the scope. Probably copy pasting the code from the project, but failed to notice this bit :).
@Override
public void save(Contact contact) {
Session session = sessionFactory.openSession ();
session.beginTransaction();
session.save(category); // should use contact here instead of category
session.getTransaction().commit();
session.close();
}
Anyway, the 3rd task is simply creating a method in the service layer which will just call the save method in your dao layer, using the same parameter which was passed to the service layer method. Sort of like adding a bridge to the dao layer, or exposing your dao layer save method in your service layer. So in your ContactServiceImpl class, just add this method.
@Override
public void save(Contact contact) {
contactDao.save(contact);
}
Note that you have a contactDao member variable already injected in the class here through @Autowired, so this is nothing else but simply exposing the dao layer save method through the service layer as I said above. This service layer method will be called by our controller layer, or maybe an Android app even if we have one to complement our web app. Which is one of the reasons why a service layer exists, cause it might seem redundant at first as it was discussed in other topics related to this course.
The save method in the dao layer btw was already created in the 2nd task (the one I pasted first where you need to correct the parameter passed to session.save()).
And not sure, but maybe you are failing the 3rd task since 2nd one isn't correct, but somehow it managed to pass. Anyway, hope I helped with understanding the specific task and the broader concepts of the controller - service - DAO layer architecture introduced in this course :).
Harrison Cassedy
Java Web Development Techdegree Graduate 12,031 PointsHarrison Cassedy
Java Web Development Techdegree Graduate 12,031 Pointsfigured it out a few hours ago thank you for the feedback tho! I'm sure someone will google this oen day and see the advice you left :)