Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial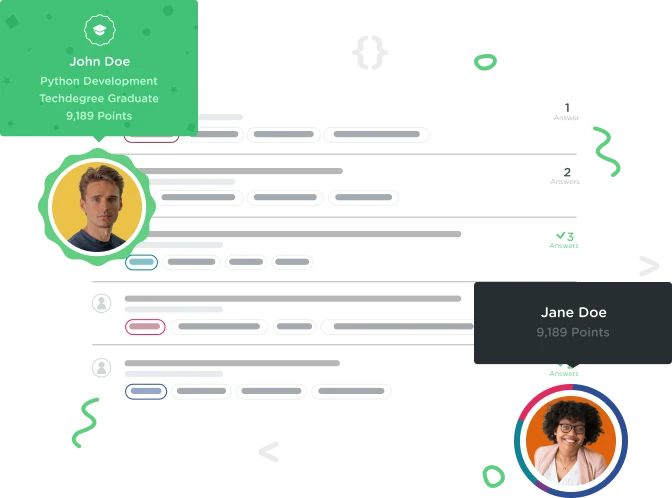

Anik Devaughn
7,751 PointsChallenge Task : Using the reduce method, extract all the customer hobbies into their own array. Store the hobbies
Hi, The Challenge ask for reduce method, and I tried using both map and reduce methods. Can I get some HINT what and where I have made mistakes with this code, Thanks in Advance!!! My code: const customers = [ { name: "Tyrone", personal: { age: 33, hobbies: ["Bicycling", "Camping"] } }, { name: "Elizabeth", personal: { age: 25, hobbies: ["Guitar", "Reading", "Gardening"] } }, { name: "Penny", personal: { age: 36, hobbies: ["Comics", "Chess", "Legos"] } } ]; let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"] // Write your code below hobbies = customers .map(customer => customer.hobbies.map(hobby => hobby.hobbies)) .reduce ((arr, hobbies) => [...arr, ...hobbies], []); //console.log(hobbies);
const customers = [
{
name: "Tyrone",
personal: {
age: 33,
hobbies: ["Bicycling", "Camping"]
}
},
{
name: "Elizabeth",
personal: {
age: 25,
hobbies: ["Guitar", "Reading", "Gardening"]
}
},
{
name: "Penny",
personal: {
age: 36,
hobbies: ["Comics", "Chess", "Legos"]
}
}
];
let hobbies;
// hobbies should be: ["Bicycling", "Camping", "Guitar", "Reading", "Gardening", "Comics", "Chess", "Legos"]
// Write your code below
hobbies = customers
.map(customer => customer.hobbies.map(hobby => hobby.hobbies))
.reduce ((arr, hobbies) => [...arr, ...hobbies], []);
//console.log(hobbies);
7 Answers
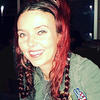
Juliette Tworsey
Front End Web Development Techdegree Graduate 32,425 PointsHi Anik!
In case you haven't solved this yet:
You are really close. There was one thing that you missed was when you were attempting to iterate over to the embedded hobbies array: you first need to get to the personal key/property before you can traverse further down into the embedded hobbies property/array.
Your map method should look like this:
.map(customer => customer.personal.hobbies.map(hobby => hobby))
Than you can initiate the reduce method like so:
.reduce((arr, hobbies) => [ ...arr, ...hobbies], [] );
I hope that this helps. This one had me stumped for a bit too.
Cheers:-)

pcachia
14,454 PointsThank you Juliette,
This was a tricky one for me as well, than after checking out the code I noticed that the results are in an array, inside an array, inside another array :O
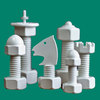
Steven Parker
231,268 PointsActually the hobbies are in an array that is inside an object that is inside another array.
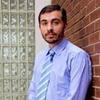
Zach Freitag
20,341 PointsThanks Juliette!
i didn't think to map before using the reduce() method.

Christine Treacy
Full Stack JavaScript Techdegree Graduate 18,412 PointsThanks Juliette! I definitely did not notice the personal key/property stuck in there. Very tricky!

chelseacheevers
13,786 PointsHi Juliette! I know this was answered a long time ago so not sure if you're still around but I was wondering! This was my initial code:
hobbies = customers
.map(user => user.personal.map(hobby => hobby.hobbies))
.reduce((arr, allHobbies) => [...arr, ...allHobbies], []);
I tried to follow what he had been doing in the previous video, which seemed closer to that (accessing person, then using map to access hobbies). Is it bc he was accessing an object in an array and I am accessing an array within an object? Thankful for your answer as I would have been stuck! But would appreciate some insight if you (or anyone!) has the time and energy to educate!
Thanks again!

Mary Hawley
Front End Web Development Techdegree Graduate 34,349 PointsI had to read the MDN docs on array.prototype.reduce() to figure out the answer for this problem. There is a relevant section labeled "Bonding arrays contained in an array of objects using the spread operator and initialValue". The documentation can be found here https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce?v=a
If reduce is written like this, it can't find "hobbies":
.reduce ((arr, hobbies) => [...arr, ...hobbies], []);
Instead, write the reduce method like this:
.reduce((prev, curr) => [...prev, ...curr.personal.hobbies], []);
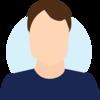
Mike Hatch
14,940 PointsThanks, Mary Hawley. I had the answer similar to your first option. It makes sense that the code in your second option is the solution. Otherwise, .hobbies
can't be accessed. All of the Challenges in this course were quite brain-draining! Will take some time to really wrap my head around these array methods, especially with .reduce
.
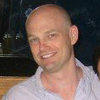
Noah Yasskin
23,947 Pointshobbies = customers
.map(customer => customer.personal.hobbies.map(hobby => hobby))
.reduce((arr, hobbies) => [...arr, ...hobbies], []);
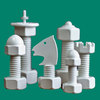
Steven Parker
231,268 PointsThe nested "map" functions aren't necessary (and causing syntax errors). Much like the previous challenge, the "reduce" by itself is enough to solve the challenge, but this time you need to extract the correct properties from each item.
I'll bet you can get it now without an explicit spoiler.

Henry Blandon
Full Stack JavaScript Techdegree Graduate 21,521 PointsThe task ask you to use the reduce method. Here is how I solved it.
hobbies = customers.reduce((accumulator, currentValue)=> {
return [...accumulator, ...currentValue.personal.hobbies]
}, []);
Here is the link to the documentation I use to solve it. (https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce#bonding_arrays_contained_in_an_array_of_objects_using_the_spread_operator_and_initialvalue)
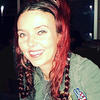
Juliette Tworsey
Front End Web Development Techdegree Graduate 32,425 PointsHi Henry,
Nice! Off topic, but there is also a nice explanation on how to remove duplicate items in an array in the link that you have posted.
Thanks:-)
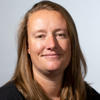
Sarah Krabacher
Full Stack JavaScript Techdegree Graduate 16,097 PointsWhere did folks learn about using the ellipsis prior to acc and curr? I attempted to write my solution as
hobbies = customers.reduce((acc, curr) => [acc, curr.personal.hobbies], []);
I don't remember seeing anything in the instructional videos that mentions ellipsis. I'm wondering if I missed something? If anyone has a good resource for explaining the use of ...
in this context, please share! I did a little bit of googling but struggled to find a resource that helped me understand.
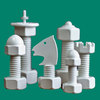
Steven Parker
231,268 PointsDepending on where they are used, ellipses might be for Rest parameters or the Spread syntax. The colored words link to the associated MDN pages on each.
Also, the Treehouse course Introducing ES2015 includes a video lesson on Rest Parameters and Spread Operator.
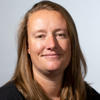
Sarah Krabacher
Full Stack JavaScript Techdegree Graduate 16,097 PointsThank you for the Treehouse video link Steven Parker ! Much appreciated :) As I continued through the course, I ended up watching a video that came AFTER the challenge and used spread syntax: https://teamtreehouse.com/library/nested-data-and-additional-exploration
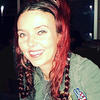
Juliette Tworsey
Front End Web Development Techdegree Graduate 32,425 PointsHi Chelsea!
When I run this code:
hobbies = customers.map(user => user.personal.map(hobby => hobby.hobbies))
.reduce((arr, allHobbies) => [...arr, ...allHobbies], []);
...I get this error:
TypeError: user.personal.map is not a function
What this is trying to say is that the map
function` will not map over an object (in this case, user.personal is an object) directly. The map method is meant to iterate over arrays, so in order to iterate over the hobbies field, try accessing the field with a more direct approach like so:
hobbies = customers.map(user => user.personal.hobbies.map(hobby => hobby))
.reduce((arr, allHobbies) => [...arr, ...allHobbies], []);
Notice that I mapped over user.personal.hobbies
(because hobbies is an array) & I also removed hobbies
from map(hobby => hobby.hobbies))
. The main thing to remember is that the map method is meant to work with arrays.
I hope that this helps and makes sense.
Cheers:-)
Manish Giri
16,266 PointsManish Giri
16,266 PointsIn my
.reduce()
, I tried it with.concat()
instead of array destructuring, and it worked. Maybe try that.