Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial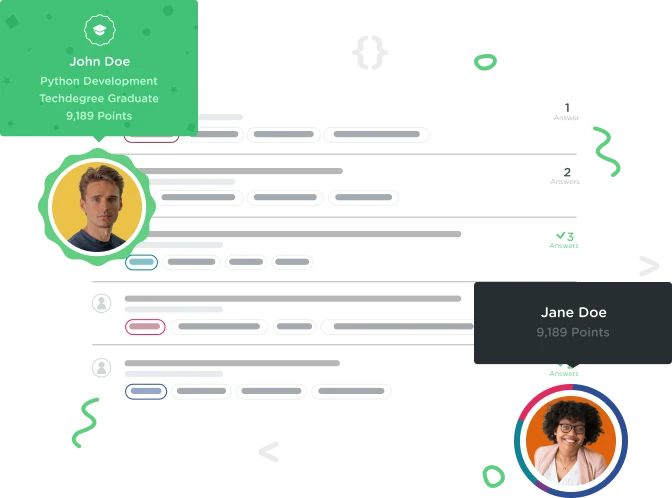

Christian Araya
2,761 PointsChallenge Tasks: Step 2 and 3 of 3
Hello could use help from some talented and experienced objective c wizards out there =)
In step 1 I'm asked to "Declare a float array named real_numbers that will hold 5 float numbers." So I enter
float real_numbers[5];
Step 1 passed. Huzzah!
Step 2 I'm asked "Using the printf function print the size in bytes of the variable real_numbers. Your output should look like the following: Array real_numbers is x bytes." To which I respond with
float real_numbers[5]; printf("Array real_numbers is %ld bytes", sizeof(real_numbers));
Step 2 passed. Woohoo!
Step 3 I'm asked "Print the length of the array real_numbers using the sizeof() function. Your output should look like the following: Array real_numbers can store x items." So I enter
float real_numbers[5]; printf("Array real_numbers can store %ld items", sizeof(real_numbers));
Output error message says that item 2 is no longer passing.
What am I doing wrong? I tried sizeof(float) but no go either. Your help is much appreciated.
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Christian,
I think you potentially have 2 problems here.
For step 3 it looks like you're not keeping your printf statement from task 2 but instead recycling it for use in step 3. You always want to keep adding to your existing code unless the instructions say otherwise. When the challenge engine is checking task 3 it also makes sure the previous tasks are still passing. So you should leave the task 2 printf in place and make a copy of it for task 3 and change the output string.
Secondly, you're not passing the correct value to printf. Task 3 wants you to figure out the length of the array. sizeof (real_numbers)
is not the length of the array. It's the total size of that array. So it's 5 * sizeof (float).
sizeof(real_numbers) / sizeof (float)
would get you the length of the array.

Christian Araya
2,761 PointsThat makes sense Jason, thanks!
I didn't know that floats had a general byte size of 4. This kept me from dividing the total number of bytes in the array by total general size of a float.
That helped so much

Jason Anello
Courses Plus Student 94,610 PointsYou're welcome.
While a float may be 4 bytes right now with current architecture it might not always be 4 bytes. I think one of the things that they want you to get from this challenge is that floats do take up some number of bytes and you should write your code in a way that is independent of what that size is.
While we might be able to pass the challenge with sizeof(real_numbers) / 4
it's only going to work if run on systems where a float is 4 bytes. It will fail if it is run on a system where a float is maybe 8 bytes.
So when you divide by sizeof (float)
it doesn't really matter what a float is. It's always going to work wherever it's run.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsIt might make more sense to work with numbers.
Floats take up 4 bytes.
sizeof (float)
would return 4.Since the array has enough space for 5 floats
sizeof (real_numbers)
would return 5 * 4 or 20. So the array takes up 20 bytes of memory.If we want to get back the length now, we can take the total size of it (20 bytes) and divide by the size of each item (4 bytes).
20 / 4 = 5 and that's how many items there are.