Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial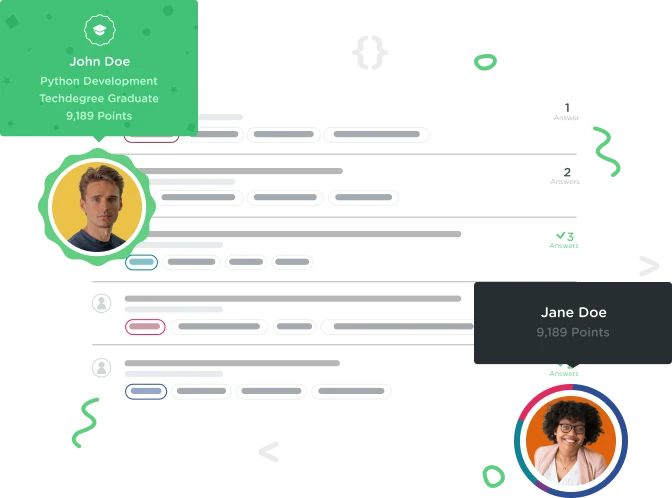
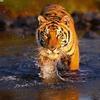
Konrad Pilch
2,435 PointsChallenge with email PHP, JS
Hi, i cant get my email working .
Heres the code:
<!-- Contact -->
<div class="object contact backgroundImg" id="contact">
<div class="container">
<div class="heading-contact">
<h1>GET IN TOUCH</h1>
</div><!-- /heading -->
<p>I will get back to you in maximum 36 hours.</p>
<form>
<div class="row">
<div class="col-xs-12 col-md-4">
<div class="form-group">
<input type="text" name="name" id="name" required class="form-control" placeholder="Your Name*">
</div>
</div>
<div class="col-xs-12 col-md-4">
<div class="form-group">
<input type="email" name="email" id="email" required class="form-control" placeholder="Your Email*">
</div>
</div>
<div class="col-xs-12 col-md-4">
<div class="form-group">
<input type="text" name="name" id="subject" class="form-control" placeholder="Your Subject (optional)">
</div>
</div>
</div><!-- /row -->
<div class="row">
<div class="col-xs-12">
<div class="form-group" id="messageContact">
<textarea name="message" id="message" required class="form-control" placeholder="Your Message*"></textarea>
</div>
</div>
</div>
<div class="btnContact pull-right">
<button type="submit" class="btn btn-warning custom-button buttonContact">SEND MESSAGE</button>
</div>
</form>
</div><!-- /container -->
</div><!-- /contact -->
<?php
// Only process POST reqeusts.
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Get the form fields and remove whitespace.
$name = strip_tags(trim($_POST["name"]));
$name = str_replace(array("\r","\n"),array(" "," "),$name);
$email = filter_var(trim($_POST["email"]), FILTER_SANITIZE_EMAIL);
$message = trim($_POST["message"]);
// Check that data was sent to the mailer.
if ( empty($name) OR empty($message) OR !filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Set a 400 (bad request) response code and exit.
http_response_code(400);
echo "Oops! There was a problem with your submission. Please complete the form and try again.";
exit;
}
// Set the recipient email address.
// FIXME: Update this to your desired email address.
$recipient = "hello@example.com";
// Set the email subject.
$subject = "New contact from $name";
// Build the email content.
$email_content = "Name: $name\n";
$email_content .= "Email: $email\n\n";
$email_content .= "Message:\n$message\n";
// Build the email headers.
$email_headers = "From: $name <$email>";
// Send the email.
if (mail($recipient, $subject, $email_content, $email_headers)) {
// Set a 200 (okay) response code.
http_response_code(200);
echo "Thank You! Your message has been sent.";
} else {
// Set a 500 (internal server error) response code.
http_response_code(500);
echo "Oops! Something went wrong and we couldn't send your message.";
}
} else {
// Not a POST request, set a 403 (forbidden) response code.
http_response_code(403);
echo "There was a problem with your submission, please try again.";
}
?>
$(function() {
// Get the form.
var form = $('#ajax-contact');
// Get the messages div.
var formMessages = $('#form-messages');
// TODO: The rest of the code will go here...
});
// Set up an event listener for the contact form.
$(form).submit(function(event) {
// Stop the browser from submitting the form.
event.preventDefault();
// TODO
});
// Serialize the form data.
var formData = $(form).serialize();
// Submit the form using AJAX.
$.ajax({
type: 'POST',
url: $(form).attr('action'),
data: formData
})
.done(function(response) {
// Make sure that the formMessages div has the 'success' class.
$(formMessages).removeClass('error');
$(formMessages).addClass('success');
// Set the message text.
$(formMessages).text(response);
// Clear the form.
$('#name').val('');
$('#email').val('');
$('#message').val('');
})
.fail(function(data) {
// Make sure that the formMessages div has the 'error' class.
$(formMessages).removeClass('success');
$(formMessages).addClass('error');
// Set the message text.
if (data.responseText !== '') {
$(formMessages).text(data.responseText);
} else {
$(formMessages).text('Oops! An error occured and your message could not be sent.');
}
});
Notes : http://blog.teamtreehouse.com/create-ajax-contact-form
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsJust noticed that your JS/jQuery is assigning the incorrect element as the form
variable:
var form = $('#ajax-contact');
In your HTML, you're using a div
with the ID of contact
, which has a descendant form
element.
So you'll either need to change the jQuery selector:
var form = $('#contact').find('form');
// OR
// var form = $('#contact form');
Or just change the HTML so the form
tag has the ID of ajax-contact
:
<div class="object contact backgroundImg" id="contact">
<div class="container">
<div class="heading-contact">
<h1>GET IN TOUCH</h1>
</div><!-- /heading -->
<p>I will get back to you in maximum 36 hours.</p>
<form id="ajax-contact">
See how you go with that.
Also, the blog article you shared says the following:
Building the HTML Form
Your first task is to set up the HTML form that will collect data from the user. Give your <
form
> element the IDajax-contact
, set themethod
attribute topost
, and theaction
attribute tomailer.php
.
<form id="ajax-contact" method="post" action="mailer.php">
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsCan you provide more information about any specific errors you're getting?