Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial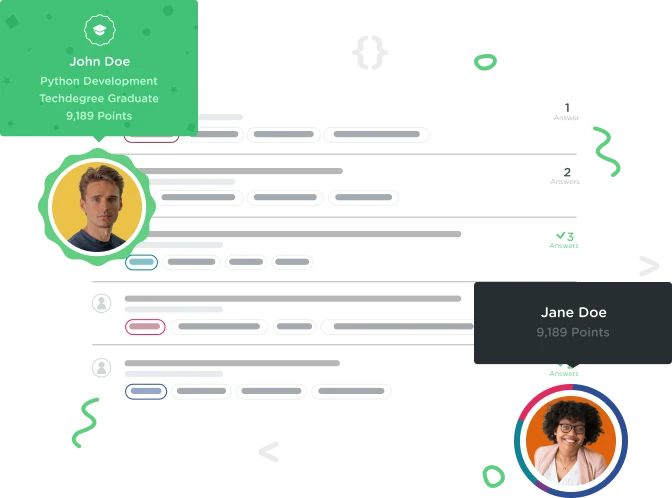

Andrew McLane
3,385 Pointschance scoring
I believe there is probably an easier way to do this, but could I get an explanation why this method doesn't work?
Thanks!
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def score_twos(self, hand):
return sum(hand.twos)
def score_threes(self, hand):
return sum(hand.twos)
def score_fours(self,hand):
return sum(hand.fours)
def score_fives(self, hand):
return sum(hand.fives)
def score_sixes(self, hand):
return sum(hand.sixes)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
def score_yatzy(self, hand):
if score_ones(5) == 5 or score_twos(5) == 10 or score_threes(5) ==15 or score_fours(5) ==20 or
score_fives(5) == 25 or score_sixes(5) == 30:
return 50
else:
return 0
1 Answer

Boban Talevski
24,793 PointsI've been asked to answer the question, so I'll do my best given that I don't really remember the rules of the game yatzy (the course was the first time I ever heard of it) and there are some things to be assumed as the challenge doesn't really explain everything clearly. What type of variable is hand? I assume it's a list. And as far as I can remember, there were five dice rolled, so I assume it contains 5 integers with the rolled dice.
Assuming this, I tried the challenge with this (don't remember how I initially solved it):
def score_yatzy(self, hand):
if hand[0] == hand[1] and hand[1] == hand[2] and hand[2] == hand[3] and hand[3] == hand[4]:
return 50
else:
return 0
And it passed.
Now why yours doesn't pass, I can only guess. First of all, you are calling score_ones, score_twos etc methods without self prepended, should be self.score_ones, self.score_twos etc. The other thing is that you have a typo in the method score_threes, you are returning sum(hand.twos) and I guess it should be hand.threes. Another thing is you are passing 5 when calling these methods, I assume you should pass hand.
But I still don't really know how these methods are supposed to work as that's not explained. What are the variables hand.ones, hand.twos etc supposed to contain? If they are supposed to contain "sublists" with the said numbers in the respective roll, your logic should work with the corrections I mentioned above. But I kinda gave it a try and it didn't seem to work.
Check it out and see if you can get it working the way you started. If not, it's best if you could tell me what are the methods and variables I mentioned supposed to do? It was most likely covered in videos and challenges leading to this one.