Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial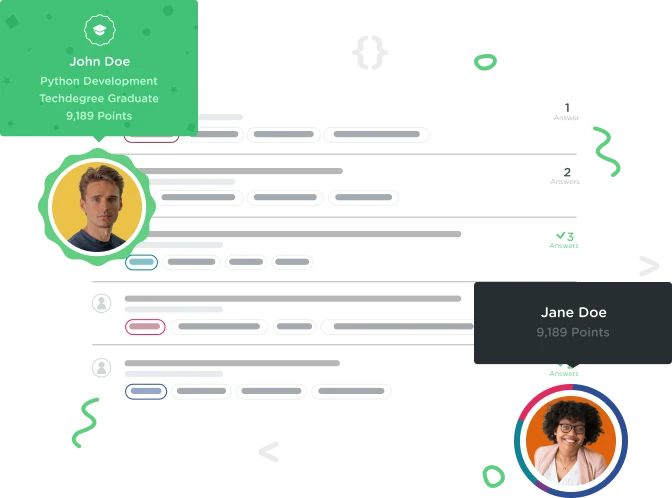
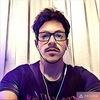
Giovanni Dalla Rizza
10,637 Pointschange a var content
during this lesson we saw an example of for loop, this is the code: /* var html = ' ';
for ( var i =1; i<=10; i+=1) { html += '<div>' + i + '</div>'; } document.write(html); */
The result was a series of number from 1 to 10. If I have to write this program I would put the document.write(html); inside the for loop and not outside like they did. Didn't understand the meaning of the program.
Thanks for helping me
3 Answers
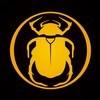
rydavim
18,814 PointsIf I understand you're question correctly, you're wondering why you couldn't do the following. If that is not the case, please elaborate.
var html = ' ';
for ( var i =1; i<=10; i+=1) {
document.write(i);
}
While this code would run, it would not produce the same result. In this case, you are overwriting the document each time you loop through. So in this case, you would only see one number on the page.
Basically, document.write()
changes the whole page. In order to see all of the numbers, you need to store them in a variable so you can write them all to the document once the loop has finished.
Hopefully that helps clarify things, but let me know if you still have questions. Happy coding! :)

Anjali Pasupathy
28,883 PointsThis is the code in the lesson:
var html = ' ';
for ( var i =1; i<=10; i+=1) {
html += '<div>' + i + '</div>';
}
document.write(html);
What this code does, is it loops through the numbers 1 to 10 and adds a div element containing the number to the html string.
// After the first loop:
html = '<div>1</div>';
// After the second loop:
html = '<div>1</div><div>2</div>';
// And so on....
// After the 10th loop:
html = '<div>1</div><div>2</div><div>3</div><div>4</div><div>5</div><div>6</div><div>7</div><div>8</div><div>9</div><div>10</div>';
After this html string is constructed, it's written to the document - thus, you see the numbers 1 to 10 in their own div elements in the document.
/*
I'm going to use [ ] to represent a div.
What the document looks like:
[ 1 ] [ 2 ] [ 3 ] [ 4 ] [ 5 ] [ 6 ] [ 7 ] [ 8 ] [ 9 ] [ 10 ]
*/
If you put "document.write(html)" inside the loop, you're writing html to the document after each modification.
// FIRST LOOP
html = '<div>1</div>';
document.write(html);
/*
What the document looks like:
[ 1 ]
*/
// SECOND LOOP
html = '<div>1</div><div>2</div>';
document.write(html);
/*
What the document looks like:
[ 1 ] [ 1 ] [ 2 ]
*/
// THIRD LOOP
html = '<div>1</div><div>2</div><div>3</div>';
document.write(html);
/*
What the document looks like:
[ 1 ] [ 1 ] [ 2 ] [ 1 ] [ 2 ] [ 3 ]
*/
// FOURTH LOOP
html = '<div>1</div><div>2</div><div>3</div><div>4</div>';
document.write(html);
/*
What the document looks like:
[ 1 ] [ 1 ] [ 2 ] [ 1 ] [ 2 ] [ 3 ] [ 1 ] [ 2 ] [ 3 ] [ 4 ]
*/
As you can see, this doesn't do the same thing as the original for loop.
If you wanted to put "document.write(html)" in the for loop without changing how the original for loop functions, you'd have to overwrite html with each new div, rather than appending each new div to html:
var html = ' ';
for ( var i =1; i<=10; i+=1) {
html = '<div>' + i + '</div>';
document.write(html);
}
I hope this helps!
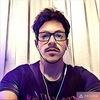
Giovanni Dalla Rizza
10,637 PointsI don't understand why var html after the loop is html = '<div>1</div><div>2</div><div>3</div><div>4</div><div>5</div><div>6</div><div>7</div><div>8</div><div>9</div><div>10</div>';
and not only html='<div>10</div>
I know that if I add something to a variable, the variable content change totally. Confusion.....

Anjali Pasupathy
28,883 PointsThis is because var html is a string. When you add one string to another string, you're doing string concatenation. That means that you're taking the two strings you're adding and putting them together.
var greeting = "Hello";
greeting += " world!";
/*
The second line is equivalent to saying greeting = greeting + " world";
This takes greeting, which has been set to "Hello", and concatenates it with the string " world!"
The resulting string stored in greeting is "Hello world!"
*/
In the example given in the video, the code inside the for loop is also doing string concatenation:
html += '<div>' + i + '</div>';
html = html + '<div>' + i + '</div>';
// THESE TWO LINES OF CODE ARE EQUIVALENT
This is a breakdown of what the code is doing in each iteration of the loop:
// FIRST LOOP
html += '<div>1</div>';
/*
What the code is doing:
html = html + '<div>1</div>';
html = '' + '<div>1</div>';
html = '<div>1</div>';
*/
// SECOND LOOP
html += '<div>2</div>';
/*
What the code is doing:
html = html + '<div>2</div>';
html = '<div>1</div>' + '<div>2</div>';
html = '<div>1</div><div>2</div>';
*/
// THIRD LOOP
html += '<div>3</div>';
/*
What the code is doing:
html = html + '<div>3</div>';
html = '<div>1</div><div>2</div>' + '<div>3</div>';
html = '<div>1</div><div>2</div><div>3</div>';
*/
// FOURTH LOOP
html += '<div>4</div>';
/*
What the code is doing:
html = html + '<div>4</div>';
html = '<div>1</div><div>2</div><div>3</div>' + '<div>4</div>';
html = '<div>1</div><div>2</div><div>3</div><div>4</div>';
*/
// AND SO ON...
// TENTH LOOP
html += '<div>10</div>';
/*
What the code is doing:
html = html + '<div>10</div>';
html = '<div>1</div><div>2</div><div>3</div><div>4</div><div>5</div><div>6</div><div>7</div><div>8</div><div>9</div>' + '<div>10</div>';
html = '<div>1</div><div>2</div><div>3</div><div>4</div><div>5</div><div>6</div><div>7</div><div>8</div><div>9</div><div>10</div>';
*/
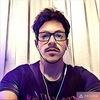
Giovanni Dalla Rizza
10,637 PointsPerfect!! In my confusion I didn't notice that it was html +=
and not html =
Thank you so much Anjali, you are great!

Anjali Pasupathy
28,883 PointsYou're welcome!