Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial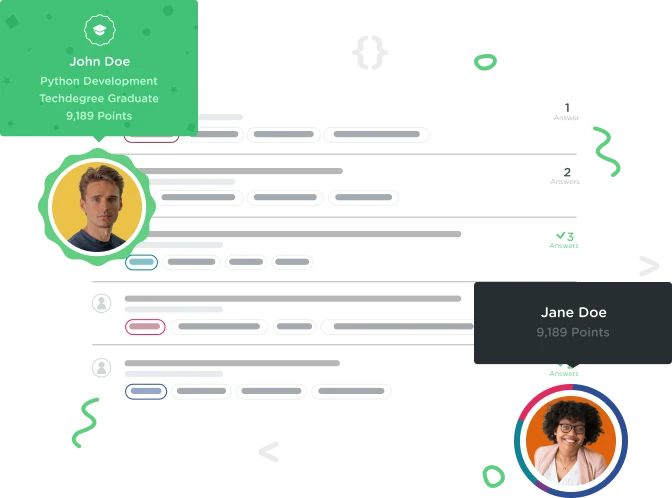
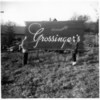
noah finnerman
15,380 PointsChange the query to a prepared statement that binds the passed argument before executing the query. this should work
This should be working, but I can' figure out why it's not. I check the docs and my syntax looks right.
<?php
function get_member($id) {
include("connection.php");
try {
$results = $db->prepare(
"SELECT member_id, email, fullname, level
FROM members WHERE member_id = ?"
);
} catch (Exception $e) {
echo "bad query";
}
$results->bindParam(1, $id, PDO::PARAM_INT);
return $results->execute();
}
4 Answers
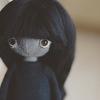
Asma Al-Marrikhi
45,525 Points<?php
function get_member($member_id) {
include("connection.php");
try {
// 1. change query to prepare
$results = $db->prepare(
"SELECT member_id, email, fullname, level
FROM members WHERE member_id = ?" // 2. change member_id = ?
);
// 3. add these two lines
$results->bindParam(1,$member_id,PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "bad query";
}
$members = $results->fetchAll();
return $members;
}

Jason Anello
Courses Plus Student 94,610 PointsHi Noah,
You replaced the original last 2 lines of code with the bindParam and execute statements.
You should move those 2 statements into the try block and leave the original last 2 lines of code unmodified.
// don't change these lines
$members = $results->fetchAll();
return $members;
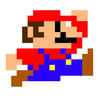
thimoschroeder
23,470 PointsThe solution is quite straight forward:
<?php
function get_member($member_id) {
include("connection.php");
try {
$results = $db->query(
"SELECT member_id, email, fullname, level
FROM members WHERE member_id = ?"
);
$results->bindParam(1, $member_id);
$results->Execute();
} catch (Exception $e) {
echo "bad query";
}
$members = $results->fetchAll();
return $members; }
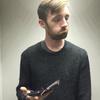
Tomas Spencer
Courses Plus Student 16,018 PointsThis is a learning community, saying something is 'quite straightforward' is only the case if you understand it. People learning, by definition, may not.
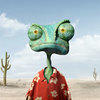
Dillon Kavanagh
14,296 PointsIt's odd, the challenge asks for the query method to be changed to use prepare rather than query. Yet as soon as you do that it reverts to stating "Oops! It looks like Task 2 is no longer passing." Any advice on this one?.
<?php
function get_member($member_id) {
include("connection.php");
try {
$results = $db->prepare(
"SELECT member_id, email, fullname, level
FROM members
WHERE member_id = " . $member_id
);
$results->bindParam(1,$member_id,PDO::PARAM_INT);
$results->execute();
} catch (Exception $e) {
echo "bad query";
}
$members = $results->fetchAll();
return $members;
}
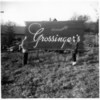
noah finnerman
15,380 PointsI am not sure why it says that Task 2 is no longer passing, but it looks like in your try block you need to put the ? as placeholder inside the quotes, instead of having .$memeber_id at the end of query string.