Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial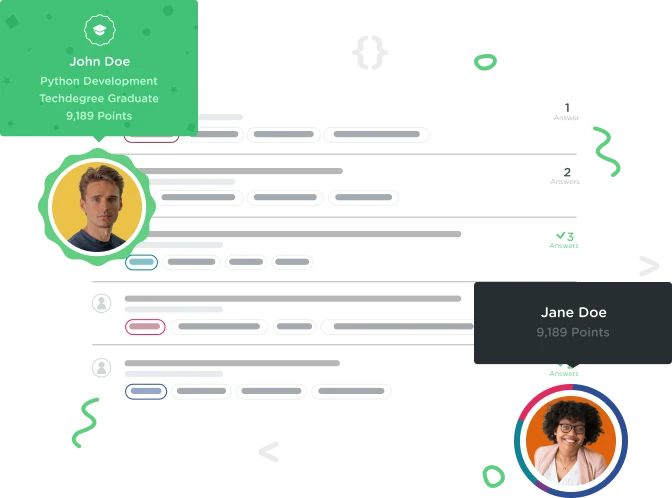
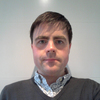
James Pask
13,600 PointsChange the size of paragraph text at different breakpoints in Bootstrap 4.
Hi everyone
I'll try to explain this the best I can. I have this code:
<div class="bg-green container rounded">
<div class="row my-4">
<div class="col-md-6 text-green px-4 p-3 col-lg-6 col-xl-6">
<h3 class="pb-2">Product 1</h3>
<p>Cras justo odio, dapibus ac facilisis in, egestas eget quam. Donec id elit non mi porta gravida at eget metus. Nullam id dolor id nibh ultricies vehicula ut id elit.</p>
<div class="row pt-5 pt-xl-5">
<div class="btn-group mx-auto" role="group" aria-label="Basic example">
<button type="button" class="btn btn-outline-light bg-green">More Info</button>
<button type="button" class="btn btn-lg">Add to Basket</button>
</div>
</div>
</div>
<div class="col-md-6 py-3 col-xl-6">
<img src="http://pinegrow.com/placeholders/img10.jpg" class="img-fluid rounded d-none d-block">
</div>
</div>
</div>
As you can see, it is bootstrapped. At the XL breakpoint the paragraph text is too small so I want to make that a bit bigger, at the MD breakpoint, the text is too large and pushes the "more info" and "add to Basket" buttons down below the line of the image. Everything displays perfectly at the LG breakpoint.
I did find in the Bootstrap documentation here (https://getbootstrap.com/docs/4.0/content/typography/) that typography isn't responsive by default but that you could easily add in the additional text into the CSS (which I've done in my style.css file):
html {
font-size: 1rem;
}
@include media-breakpoint-up(sm) {
html {
font-size: 1.2rem;
}
}
@include media-breakpoint-up(md) {
html {
font-size: 1.4rem;
}
}
@include media-breakpoint-up(lg) {
html {
font-size: 1.6rem;
}
@include media-breakpoint-up(xl) {
html {
font-size: 1.6rem;
}
}
}
This isn't working. I've googled and it says something about @include tags being part of SASS but I haven't got round to learning that yet so I don't really know what that's on about. I also thought that the best way to override text was to use the body element but this is using the html element instead.
Can someone please help? Hopefully I've provided enough info. I did do screenshots but you can't attach them to the posts.
Look forward to hearing your thoughts.
2 Answers
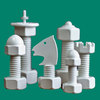
Steven Parker
230,879 PointsIf you don't use a Sass pre-compiler you just need to translate this into plain CSS:
/* Extra small devices (portrait phones, less than 576px) */
/* No media query since this is the default in Bootstrap */
html {
font-size: 1rem;
}
/* Small devices (landscape phones, 576px and up) */
@media (min-width: 576px) {
html {
font-size: 1.2rem;
}
}
/* Medium devices (tablets, 768px and up) */
@media (min-width: 768px) {
html {
font-size: 1.4rem;
}
}
/* Large devices (desktops, 992px and up) */
@media (min-width: 992px) {
html {
font-size: 1.6rem;
}
}
/* Extra large devices (large desktops, 1200px and up)
// (you don't need this one unless you want something different from LG)
@media (min-width: 1200px) {
html {
font-size: 1.6rem;
}
} */
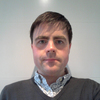
James Pask
13,600 PointsGiven the fact that I had to google what a Sass pre-compiler actually was, I'll just convert this to plain old CSS and do it that way. I wonder why they don't consider responsive typography a core part of bootstrap. Maybe its because there are less and less of us using large screen displays.