Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial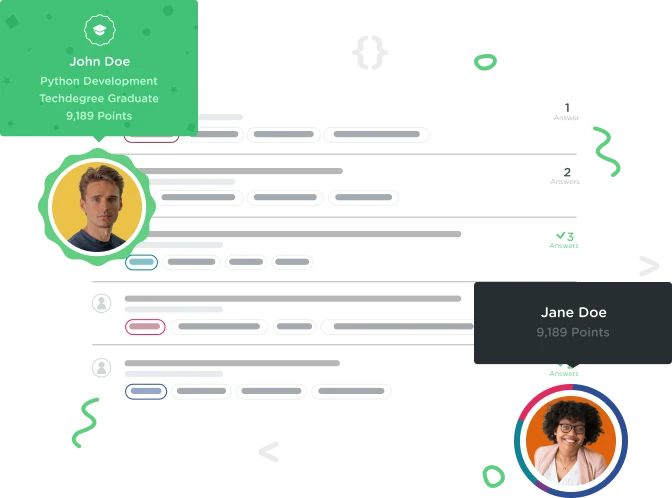

Sean Urgel
Courses Plus Student 6,650 PointsChange this into a constructor function
In the Javascript course "https://teamtreehouse.com/library/build-a-simple-dynamic-site-with-nodejs" the app JS file they gave is kind of outdated, but it works sometimes.
The old code is a constructor function so it can be called and used as const studentData = new Profile(username);
The old code is unstable as of the moment - as sometimes the util library won't read all the time.
The new code is just a normal function and cannot call "new Profile(username)"; So for my future projects - and that the code won't get deprecated can someone help me turn the new code into a constructor function?
/************************************************* Old code which is a constructor function var EventEmitter = require("events").EventEmitter; var https = require("https"); var http = require("http"); var util = require("util");
/**
- An EventEmitter to get a Treehouse students profile.
- @param username
-
@constructor */ function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json) var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) { var body = "";
if (response.statusCode !== 200) { request.abort(); //Status Code Error profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")")); } //Read the data response.on('data', function (chunk) { body += chunk; profileEmitter.emit("data", chunk); }); response.on('end', function () { if(response.statusCode === 200) { try { //Parse the data var profile = JSON.parse(body); profileEmitter.emit("end", profile); } catch (error) { profileEmitter.emit("error", error); } } }).on("error", function(error){ profileEmitter.emit("error", error); });
}); }
util.inherits( Profile, EventEmitter );
module.exports = Profile;
*****************************/ End of old code
/***************************** ES6 syntax - but not a constructor function //requre the https module const https = require('https');
//require http module const http = require('http');
const getProfile = (username, course) =>{
try{
// Connect to the teamtreehouse API URL (https://teamtreehouse.com/seanurgel.json)
const request = https.get(https://teamtreehouse.com/${username}.json
, (response) => {
if(response.statusCode === 200){
let body = "";
//Read the data
response.on('data', (d) => {
body += d.toString();
});
response.on('end', () => {
try{
//Parse the data
const profile = JSON.parse(body);
}catch(error){
console.error(`Newbies: User is not found`);
console.error(`Pros: ${error.message}`);
}
});
}else{
const message = `There was an error getting the profile ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
}).on('error', (error) => {
console.error(`Problem: ${error.message}`);
console.error(`URL link is incorrect / not found`);
});
}catch(error){
printError(error);
console.error('Lacking HTTPS');
}
}
module.exports.get = getProfile; *****************************/ end of new code