Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial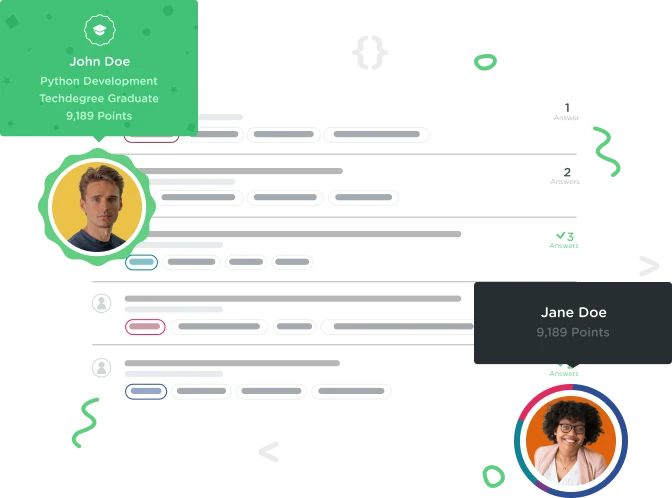
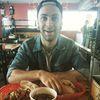
Zaq Dayton
2,244 PointsChanges in C-style for loop statement for Swift 3 explanation? The example in the video has already been depreciated...
So, first off, I hate how none of the videos so far have been updated with the new Swift 3 syntax. A lot of the stuff learned has already been depreciated and is pretty confusing for someone just starting to learn Swift.
Anyway, this C-style code for my "for" loop has been depreciated:
for var i = 0; i < todo.count; i++ {
// loop stuff
}
At first, I was thinking that only the i++ needed to be replaced to look like this based off of other out-dated stuff in previous video tutorials:
for var i = 0; i < todo.count; i += 1 {
// loop stuff
}
However, the xcode editor is telling me that all of C-style has depreciated and should now look like this:
for i in 0 ..< todo.count {
// loop stuff
}
This is a completely different approach. Now it's a "for-in" loop, rather than a simple "for" loop. Can anyone explain this change to me? I don't really understand the
0 ..<
part. Also, does "i" need to be initialized prior to this loop, or does for i
create a variable named "i" within this loop's scope? Thanks for the help!
2 Answers
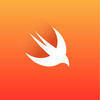
Steven Deutsch
21,046 PointsHey Zaq Dayton,
First of all I can understand your frustration regarding the changes to the Swift language. It's definitely a challenge to learn something that is not completely stable.
There are no updates to the videos for Swift 3, because Swift 3 is not even released yet. The compiler is simply giving you a warning saying that you should be weary of using this code because Swift 3 won't support it in the future.
This change to use the for-in loop is actually much simpler than the for loop. I mean honestly, just look how much cleaner the code is.
The for-in loop is used to iterate over a sequence of values. This could be an array, items in a dictionary, a string. It simply takes the first item out of the sequence and assigns it to a temporary constant for use in the loop body. The loop automatically increments itself. There is no need to define an index or an incrementor.
// This is the half-open range operator in Swift
..<
// It means that for this range we will go from the first number specified to the end number
// but NOT including the end number
0..<10 // for example, this is a range of Integers 0 to 9, NOT including 10
// Now lets take a look as to why 0..<todo.count is used
0..<todo.count
Here you are telling the loop to iterate over a range of Integers. The first being 0 and the last being one less than the count of the todo array. Since indexes are zero based, the last index of an array will always be one less than the current number of items in the array. This is why the half-open range operator is used. We don't want an index that is out of range because it will crash our code.
Good Luck

Tim Seme
1,664 Pointslet totalScore = initialScore totalScore = ++initialscore
how to make a constant change using the increment operation?
Zaq Dayton
2,244 PointsZaq Dayton
2,244 PointsThanks, Steven! I understand it much better now. I appreciate the help!
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsNo problem. Glad I could help. Let me know if you have any other questions :)