Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial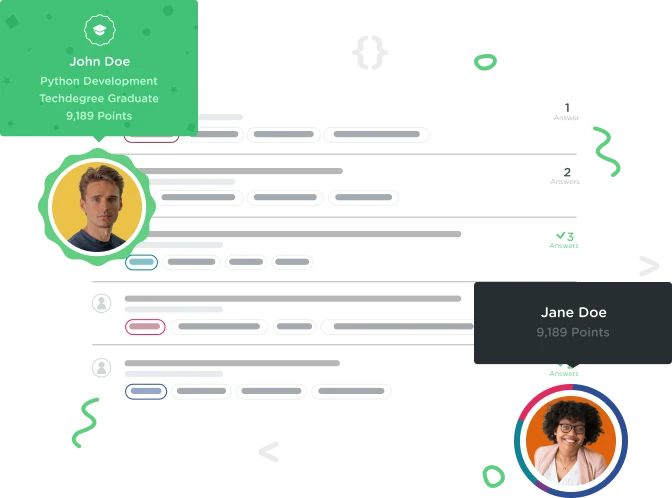

douglas gray
888 PointsChanging a pair of if statements to a single switch case statement.
Hello. The code looks like this:
<?php //Available roles: admin, editor, author, subscriber if (!isset($role)) { $role = 'subscriber'; }
//change to switch statement if ($role != 'admin') { echo "You do not have access to this page. Please contact your administrator."; }
And the directions are :
" Display separate messages based on the role of the logged in user. First, add a message for our admin and keep the current message as the default.
Change the if statement to a switch statement, keeping the current message as the default. Add a check for the role of "admin" and display this message : 'As an admin, you can add, edit, or delete any post.' "
Please offer me any solution if you are inclined. I've tried at least eight variations and the most common errors are that the wrong default option is present or that there is no case for 'admin', but neither of those errors is valid. Thank you.
<?php
//Available roles: admin, editor, author, subscriber
if (!isset($role)) {
$role = 'subscriber';
}
//change to switch statement
if ($role != 'admin') {
echo "You do not have access to this page. Please contact your administrator.";
}
1 Answer
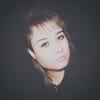
-- --
12,382 PointsHi Douglas. :)
In order to change the if statement to a switch statement, you first have to understand what's going on in the code. So, let's have a look at the code first.
The role set in the first if statement is subscriber, which means that you're looking at the output from this role. The second 'if statement' checks if the role is not (!=) 'admin', and if it's not, the code should echo "You do not have access to this page. Please contact your administrator." to the page. So, because our role is set to 'subscriber', the output will actually be this message.
Changing the 'if statement' to a 'switch statement'
A switch statement will first check the expression ($role, in this case) and then it will check if any of the case values match. So, in order to display a different message based on the role of the logged in user, we first need our code to evaluate the $role variable, like so:
switch ($role) {
}
Then, we need to state what we would like to happen for a specific role. For task 1 of the challenge, we have to add the 'admin' role and a message should be echoed to the page if the role of the logged in user is actually 'admin'. We end the code block with a break, so that it won't jump to the next case automatically.
switch ($role) {
case 'admin':
echo "As an admin, you can add, edit, or delete any post.";
break;
}
We then need to add the default case, which will be executed if none of the cases match the expression. This is done as follows:
switch ($role) {
case 'admin':
echo "As an admin, you can add, edit, or delete any post.";
break;
default:
echo: "You do not have access to this page. Please contact your administrator.";
}
You should now be able to pass task 2 by yourself. :)
Good luck! ??

douglas gray
888 PointsThank you for your specificity and attention. This didn't work, though. I originally wrote a block similar to this but with the "isset" in the "switch()" at the top. I did that bc it says to "not change the code above the switch statement." When I fix this i get a variety of messages saying that either, $role, or a case, or an echo are "unexpected. I've tried explicitly setting $role equal to admin in the first and only case, and I've tried adjusting the indentation many times but this doesn't work either.
Scott Lougheed
19,390 PointsScott Lougheed
19,390 Pointscan you show us at least one of your attempts at writing this as a switch statement? That will allow us to see where you might be making mistakes and provide better help.