Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial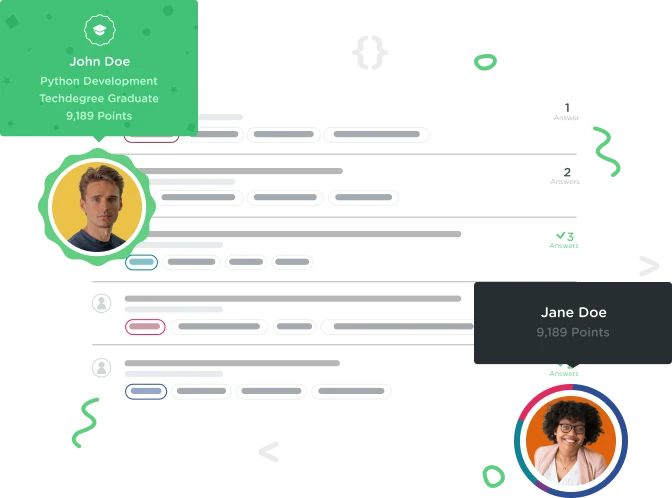

Christopher Anderson
1,763 PointsChanging a while loop, into a do while loop
I have while loop and I am supposed to change the loop into a do/while loop, however the challenge is not passing.
do{
document.write("You know the secret password. Welcome")
} while (secret !== "sesame") {
var secret = prompt("What is the secret password?");
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
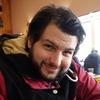
Eric M
11,545 PointsHi Christopher,
A do while loop executes a block of code, then checks the while condition. If the while condition is true, it runs the do block again, if false it continues on.
So, with that in mind, let's take a look at the do while loop you posted, ignoring everything else.
do
{
document.write("You know the secret password. Welcome")
}
while (secret !== "sesame")
This code calls document's write()
method with a string literal. Then it checks the while condition and if secret doesn't equal "sesame" it runs the loop again. This is an infinite loop that will never get to the secret prompt. (You've also left the semicolon off your document.write() call.)
Instead, you need to put the code that can change the condition from true to false within the loop, and the success message after the loop.
do
{
var secret = prompt("What is the secret password?");
}
while (secret !== "sesame")
document.write("You know the secret password. Welcome");
Let's go one step further though and move the variable declaration outside of the loop. This isn't strictly necessary for the operation of the program or passing this challenge, and in JavaScript it doesn't even have performance implications (it does in langauges that handle memory and interpretation of code differently). However, it's common JavaScript convention to declare variables outside of the loop. Following convention makes your code faster for other programmers to read, and a consistent style helps when programs are authored by many people.
var secret;
do
{
secret = prompt("What is the secret password?");
}
while (secret !== "sesame")
document.write("You know the secret password. Welcome");
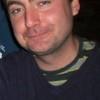
Richard Scott
25,647 PointsHi! you need to assign the variable called 'secret' inside the loop, so that the prompt always appears at least once. You can start off by declaring it as an empty string, then assign it the value when needed :) hope thats helpful!

Christopher Anderson
1,763 PointsThank you!!!
Christopher Anderson
1,763 PointsChristopher Anderson
1,763 PointsThank you!!!