Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial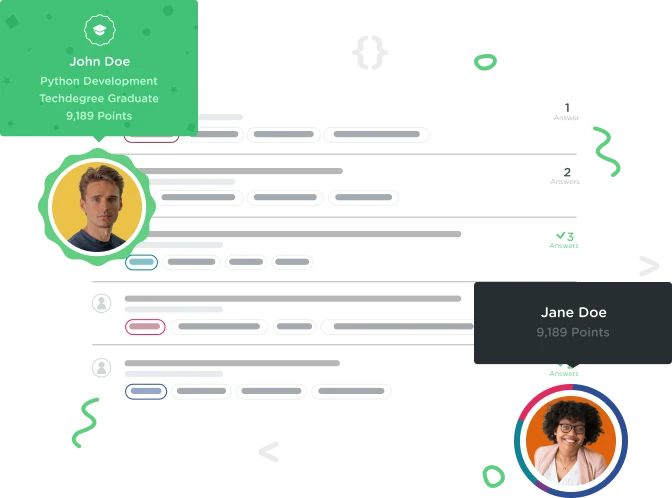
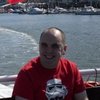
Sean Flanagan
33,235 Points"Changing Course" challenge, Task 2
Hi. This is the code I've written for Task 2.
Does anyone know where I've gone wrong please?
com/example/model/Course.java
package com.example.model;
import java.util.List;
public class Course {
private String mName;
private List<Video> mVideos;
public Course(String name, List<Video> videos) {
mName = name;
mVideos = videos;
}
public String getName() {
return mName;
}
public List<Video> getVideos() {
return mVideos;
}
}
package com.example.model;
public class Video {
private String mTitle;
public Video(String title) {
mTitle = title;
}
public String getTitle() {
return mTitle;
}
public void setTitle(String title) {
mTitle = title;
}
}
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
List<Video> videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
videoList.add(1, video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) { //My code for Task 2
videoList = course.getVideos(); //First error
Map<String, Video> videoMap = new TreeMap<>();
for (Video video : videoList) { //Second error
videoMap.put(video.getTitle(), videoList.get(1)); //Third error
}
return videoMap;
}
}
Thanks in advance. :-)
5 Answers
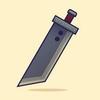
Allan Clark
10,810 Pointsyeah but you need the generic, sorry forgot to type that.
also in your put statement, video.get(video) is redundant, you already have the video, no need to get it again. The for each loop does that for you. The for-each loop is iterating through the whole videoList, the video variable you declare in the for-each statement is a place holder for each one that is pulled out.
The end product should look like this:
public Map<String, Video> videosByTitle(Course course) { //My code for Task 2
List<Video> videoList = course.getVideos();
Map<String, Video> videoMap = new TreeMap<>();
for (Video video : videoList) { //Second error
videoMap.put(video.getTitle(), video); //Third error
}
return videoMap;
}
You got it brotato, keep on coding ;)
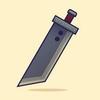
Allan Clark
10,810 PointsFirst you forgot to add the variable type when creating the videoList variable.
List<Video> videoList = course.getVideos(); //First error
That takes care of all the compile time errors. Next you have a logical error in your for-each loop.
videoMap.put(video.getTitle(), videoList.get(1));
The videoList.get(1) part stays the same for every loop. This means that you will grab the next video, get its title and associate it with whatever the video at index 1 is. We can correct this by simply changing that to just video. This will go through the whole list grabbing the video and adding it to the Map with the title as the key and video as the value.
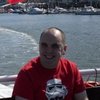
Sean Flanagan
33,235 PointsThanks Allan. That saw me through. I would mark your last answer as Best Answer but there's no option to do that underneath. I'll pick another of your answers for you.
Thank you. :-)
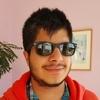
faraz
Courses Plus Student 21,474 PointsHey Sean,
I went on and made Allan's answer the Best Answer. I'm glad you found his answer helpful.
Mod Faraz
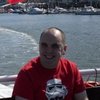
Sean Flanagan
33,235 PointsHi Allan. Thanks for your efforts to help. I'm still getting compiler errors. Here's my new syntax:
import com.example.model.Course;
import com.example.model.Video;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
public class QuickFix {
public void addForgottenVideo(Course course) {
// TODO(1): Create a new video called "The Beginning Bits"
List<Video> videoList = course.getVideos();
Video video = new Video("The Beginning Bits");
// TODO(2): Add the newly created video to the course videos as the second video.
videoList.add(1, video);
}
public void fixVideoTitle(Course course, String oldTitle, String newTitle) {
}
public Map<String, Video> videosByTitle(Course course) {
video = course.getVideos(); //First error
Map<String, Video> videoMap = new TreeMap<>();
for (Video video : videoList) { //Second error
videoMap.put(video.getTitle(), video.get(video)); //Third error
}
return videoMap;
}
}
Errors:
./QuickFix.java:24: error: cannot find symbol
video = course.getVideos(); //First error
^
symbol: variable video
location: class QuickFix
./QuickFix.java:26: error: cannot find symbol
for (Video video : videoList) { //Second error
^
symbol: variable videoList
location: class QuickFix
./QuickFix.java:27: error: cannot find symbol
videoMap.put(video.getTitle(), video.get(video)); //Third error
^
symbol: method get(Video)
location: variable video of type Video
3 errors
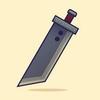
Allan Clark
10,810 PointsIt is still where you have //First error. Change that variable name back to videoList, but add "List<Video>" just before it.
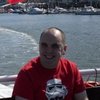
Sean Flanagan
33,235 PointsIt still won't work. When you say "add 'List' just before videoList, is this what you meant?
List videoList = course.getVideos();
Thanks Allan :-)
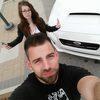
Jordan Ernst
5,121 Pointsit was a list of video>>
List<Video> videoList= course.getVideos();
Jordan Ernst
5,121 PointsJordan Ernst
5,121 Pointswhy do we add 1 in?
videoList.add(1, video);