Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial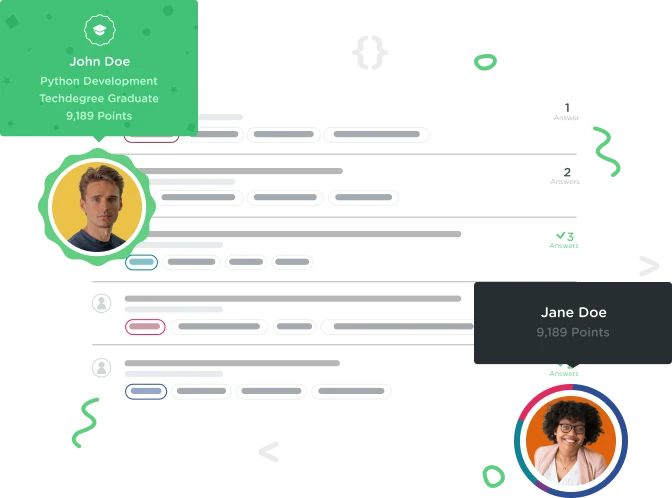
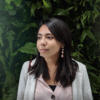
Jenn Herrarte
Front End Web Development Techdegree Student 3,118 PointsChanging Javascript List to Letters instead of Numbers
Hi all,
Can someone help change my javascript code so that list is letters instead of numbers? I would like the letters A to Z on my buttons instead of numbers 1-26. I've tried looking for different ways but I'm not sure if I should stick to a list or try something else with variables.
HTML
<ol class="list-group">
<div class="fact-modal-wrapper">
<div class="modal-card">
<div id="modal-content"></div>
<button id="closeModal_btn" onclick="closeModal()">✖</button>
</div>
</div>
JAVASCRIPT
const list = document.querySelector('.list-group');
for (i = 1; i <=26; i++) {
list.innerHTML += `<li><button>${i}</button></li>`;
}
list.addEventListener('click', (e) => {
const btns = document.querySelectorAll('.list-group li button');
const modal = document.querySelector('.fact-modal-wrapper');
const number = e.target.textContent;
document.getElementById('modal-content').innerHTML = `<h2>${number}</h2>
<p>${facts[number-1]}</p>`;
modal.classList.toggle('active');
});
function closeModal() {
document.querySelector('.fact-modal-wrapper').classList.toggle('active');
}
const facts = [
"A is for American Psycho Abaa.",
]
4 Answers
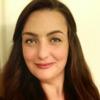
Jennifer Nordell
Treehouse TeacherHi there, Jenn Herrarte! There's a few ways to solve this. You could make an array, sure. That's one option. You could also use pretty much what you have now. There's a method on the String
class called fromCharCode
. An "a" is character code 97. So without a lot of changes to your code, you could do this:
for (i = 1; i <=26; i++) {
list.innerHTML += `<li><button>${String.fromCharCode(96 + i)}</button></li>`;
}
That will give you buttons with lower-case a-z on them. If you want uppercase you'd need to change that to (64 + i) because 65 is the ASCII code for the upper case "A".
But I think there are probably better solutions. One solution is to put the alphabet in a string and loop through the string.
const alphabet = "abcdefghijklmnopqrstuvwxyz";
for(i = 0; i < alphabet.length; i++) {
list.innerHTML += `<li><button>${alphabet[i]}</button></li>`;
}
But if I'm honest, that's better, in my opinion but still probably not the cleanest solution (or the most modern). I might instead opt for a for of
loop which does the same thing. In my opinion, it makes the code easier to follow and understand.
const alphabet = "abcdefghijklmnopqrstuvwxyz";
for(let letter of alphabet) {
list.innerHTML += `<li><button>${letter}</button></li>`;
}
Hope this sparks some inspiration!
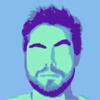
Cameron Childres
11,818 PointsHi Jenn,
I think String.fromCharCode() should work:
for (i = 1; i <=26; i++) {
list.innerHTML += `<li><button>${String.fromCharCode(64 + i)}</button></li>`;
}
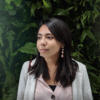
Jenn Herrarte
Front End Web Development Techdegree Student 3,118 PointsThank you Cameron!!
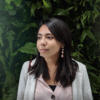
Jenn Herrarte
Front End Web Development Techdegree Student 3,118 PointsJennifer Nordell - Jennifer, Thank you so so much for your detailed response. I really appreciate how you provided different solutions with context. I'm super new to JS and writing cleaner, easy to read code is a skill I'm trying to gain.
So the first solution you provided worked, but now the button facts are 'undefined.' I think I have to change something in the rest of the JS code to 're-link' the fact with the new letter buttons. Here is the code pen I have for this project, in case seeing more of the code helps - https://codepen.io/jennnuwine/pen/bGeMrME I think I can replace this line of code: const number = e.target.textContent; but I'm not sure with what.
Thank you again!!
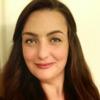
Jennifer Nordell
Treehouse TeacherHi there, Jenn Herrarte! If you keep the fromCharCode
then you are making a character from a number. Which means that your number
isn't a number anymore. It's a letter So, the way to do that would be to reverse that. You'd get the
charCodeAt
the index of 0 because the innertext there is only one letter. That will give you the character code as a number. But you will then need to subtract the amount you originally added.
Here was my solution for that part:
const list = document.querySelector('.list-group');
for (i = 1; i <=26; i++) {
list.innerHTML += `<li><button>${String.fromCharCode(64 +i )}</button></li>`;
}
list.addEventListener('click', (e) => {
const btns = document.querySelectorAll('.list-group li button');
const modal = document.querySelector('.fact-modal-wrapper');
const number = e.target.textContent.charCodeAt(0) - 64;
document.getElementById('modal-content').innerHTML = `<h2>${number}</h2>
<p>${facts[number-1]}</p>`;
modal.classList.toggle('active');
});
Hope this helps!
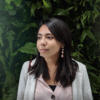
Jenn Herrarte
Front End Web Development Techdegree Student 3,118 PointsJennifer Nordell - Thank you so much again for your detailed response! It worked, but going to play around with it so I can understand the breakdown / how this all works in the code. Thank you again!!
Cameron Childres
11,818 PointsCameron Childres
11,818 PointsI'm cracking up at how often we post at the same time Jennifer Nordell š¤£
Your answers are amazing and I often learn more from them than I do from initially looking in to the question. Thanks for being so active!
EDIT: I just realized you're the person being interviewed in the Treehouse blog post that got me interested in participating in the community in the first place. So consider this a double thank you š
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherAwwwww thanks and you're welcome, Cameron Childres! You also leave really great answers