Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial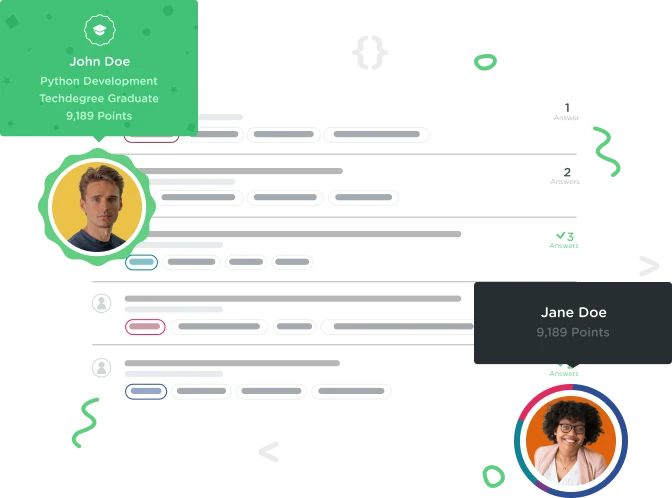

Joshua Lee
3,625 PointsChanging label text to new string within array
So I have one last piece of code for my fun facts app. The initial ViewController of my app shows UIImages of different categories that the user can click to learn new facts about. Once the user taps on the image, it takes them to the ViewController that then displays a fact from the kFacts array in my FactBook class. The second ViewController is similar to that of the "Build A Simple IOS App." Depending on what image is tapped, it shows a fact about that category. So my problem is, I created a randomFact method to display a new random fact from the specified array depending on which UIImage is tapped. When I tap the "showNewFact" button, my app crashes; is there anyway to fix this? I'll post my code if this is confusing.
3 Answers

Joshua Lee
3,625 PointsHere's my FactBook.m containing my arrays with facts.
NSString *const kTitle = @"title";
NSString *const kFacts = @"facts";
NSString *const kIcon = @"icon";
NSString *const kBackgroundColor = @"backgroundcolor";
@implementation FactBook
- (instancetype)init {
self = [super init];
if (self) {
_library = @[@{kTitle: @"Random",
kIcon: @"random.pdf",
kBackgroundColor: @{@"red": @236.0, @"green": @81.0, @"blue": @59.0, @"alpha": @1.0},
kFacts: @[@"When hippos are upset, their sweat turns red."
,@"The average woman uses her height in lipstick every 5 years."
,@"Cherophobia is the fear of fun."
,@"In the UK, it is illegal to eat mince pies on Christmas Day!"
,@"A flock of crows is known as a murder."]
},
@{kTitle: @"Dogs",
kIcon: @"dog.pdf",
kBackgroundColor: @{@"red": @236.0, @"green": @140.0, @"blue": @37.0, @"alpha": @1.0},
kFacts: @[@"Puppies have 28 teeth and normal adult dogs have 42."]
},
@{kTitle: @"Food",
kIcon: @"food.pdf",
kBackgroundColor: @{@"red": @236.0, @"green": @206.0, @"blue": @180.0, @"alpha": @1.0},
kFacts: @[@"Pringles once had a lawsuit trying to prove that they weren't really potato chips."]
},
Here's my randomFact method
```- (NSString *)randFact {
int random = arc4random_uniform((int)self.library.count);
return [self.library objectAtIndex:random];
}

Joshua Lee
3,625 PointsHere's my ViewController that displays the [0] index string within the array of whatever UIImage instance is sent over from the category page. my problem is the showNewFact method.
@implementation DisplayFactsViewController
// changes background/button to random color. Label to random fact.
- (IBAction)showNewFact {
UIColor *randomColor = [self.colorWheel randomColor];
self.view.backgroundColor = randomColor;
self.factColorButton.tintColor = randomColor;
self.funFactLabel.text = [self.factBook randFact];
}
//Library instance passed from category page, background/buttontint set to random color
- (void)viewDidLoad {
[super viewDidLoad];
self.factBook = [[FactBook alloc] init];
self.colorWheel = [[ColorWheel alloc] init];
UIColor *randomColor = self.colorWheel.randomColor;
self.view.backgroundColor = randomColor;
self.factColorButton.tintColor = randomColor;
if (self.library) {
self.funFactLabel.text = self.library.categoryFacts[0];
self.navigationItem.title = self.library.categoryTitle;
}
}

Joshua Lee
3,625 PointsHere's the category page ViewController containing all the UIImages. the prepareForSegue method sends over the UIImage instance that was tapped.
@implementation CategoryPageViewController
- (void)viewDidLoad {
[super viewDidLoad];
//Creates library instance for each image in category page
for (NSUInteger index = 0; index <self.categoryImages.count; index++ ) {
FactsLibrary *library = [[FactsLibrary alloc] initWithIndex:index];
UIImageView *categoryImageView = self.categoryImages[index];
categoryImageView.image = library.imageIcon;
categoryImageView.backgroundColor = library.backgroundColor;
}
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
}
/* Determines which image was tapped, then passes index value over to library instance
where then the correct information is displayed when segue is performed
*/
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([segue.identifier isEqualToString:@"categoryToDisplay"]) {
UIImageView *categoryImageView = (UIImageView *)[sender view];
if ([self.categoryImages containsObject:categoryImageView]) {
NSUInteger index = [self.categoryImages indexOfObject:categoryImageView];
DisplayFactsViewController *displayFactsViewController = (DisplayFactsViewController *)segue.destinationViewController;
displayFactsViewController.library = [[FactsLibrary alloc] initWithIndex:index];
}
}
}
- (IBAction)showFactPage:(id)sender {
[self performSegueWithIdentifier:@"categoryToDisplay" sender:sender];
}
@end