Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial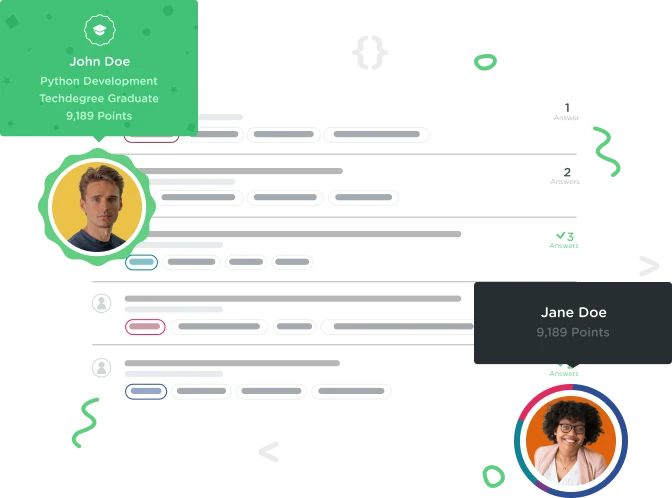

Joshua Morillo
2,562 PointsChanging state...I got it right but I'm not sure why.
Why is the phrase MAX_BARS used twice? I remember in an earlier video Craig mentioned DRY (don't repeat yourself). I got it wrong the first few tries because I trying to figure out a way to avoid using it again. Is it ok to repeat something at certain times?
Why is charge and MAX_BARS public while barCount is private? Shouldn't all three of them be public to the consumer?
Maybe I'm over thinking this, but I think programming is going to be a lot like math in that if you don't know why you got a simple answer right in the beginning, it can cause big problems in the future.
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
public void charge()
{
barCount = MAX_BARS;
}
private String color;
public GoKart(String color)
{
this.color = color;
}
public String getColor()
{
return color;
}
}
3 Answers
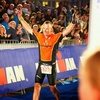
Steve Hunter
57,712 PointsHi Joshua,
You use MAX_BARS
twice, correctly. The first time you use it, you are creating the constant and putting the value 8 in it. Every subsequent time you use it, you are using the value it holds, 8. One great benefit of this is that you can change the maximum charge of every GoKart
object by altering the value in one place. So, if we decide later that GoKart
s should have a 9 bar charge, we can just change that in the single place at the start of the class where you created the constant.
The private/public thing is a little more complicated. The constant needs to be public, I think, so that any user of the class can access it. It is a class constant (Google that!) so it can be accessed right off the class, not requiring an instance. The other member variables are private so they are only accessible by using the getter and setter methods. It is considered "bad form' to be able to alter the value of member variables directly. The use of getter & setter methods is strongly encouraged.
I hope that makes some sense? Let me know if you have further queries.
Steve.

Teja Tummalapalli
5,592 PointsHi,
The MAX_BARS
is an integer being used as a constant. It an convention to declare constant as static
and final
. Refer [SO question] for more info regarding this.
Coming back to your questions.
1.MAX_BARS
is a constant, It can be used at many places but does not break DRY.
DRY specifically mean don't repeating the code. Using variables,constants or calling methods multiple times does not break DRY.
2.In general variables are declared private
. So barCount
is declared private
, but MAX_BARS
is being used as a constant here. Constants are declared public

Joshua Morillo
2,562 PointsThank you for your responses.
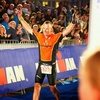
Steve Hunter
57,712 PointsNo problem!
Teja Tummalapalli
5,592 PointsTeja Tummalapalli
5,592 PointsSteve Hunter Suppose I don't have an intention to use the constant in other classes. Can I make the constant private?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Teja,
You can create a constant that is private, yes. The keyword
static
makes the variable/constant available directly from the class without needing an instance of the class. I'd guess you'd have to omit that keyword to be a private variable/constant too.If you want a constant available to every instance of the class, declare it
private final
. This means every instance will be able to carry the private member constant. That strikes me as wasteful; it is a constant - why have the same constant on every instance of the class? In this case, the constant is an integer so the overhead is minimal. But if the constant was a huge number or other large data-type, duplicating it across every instance is going to impact on performance.Having one constant as a
static
means that the class has the constant in one place only. I think, by its very nature, that must bepublic
; I can't see how it can beprivate
if it is accessible without an instance? I may be wrong and would be interested in the definitive answer. But, holding a single constant, rather than replicating it over every instance is efficient memory-wise and avoids unnecessary duplication.Make sense?
Steve.
Teja Tummalapalli
5,592 PointsTeja Tummalapalli
5,592 PointsHi, Steve Hunter . Having
static
makes sense as it avoids duplication.My understanding is even though the variable is declared as
static
it can still beprivate
. The variables likeprivate static final foo
can be used in the methods of the same class but other classes cannot access them either directly of by creating instances. They can act as private constants.I may be wrong but, In this case , as the variable
MAX_BARS
is not being used by other classes , it can instead beprivate
This is a stack overflow question I came across. Let me know what you think.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI agree. The
private static
can be seen only by instances ofClass
but it is shared by all those instances and the Class itself, i.e. they all hold the same value, indeed the same area of memory storing that value.I've not done this course but assuming the accessing of
MAX_BARS
is only required by instances ofGoKart
or byGoKart
itself, there is no reason whyMAX_BARS
cannot beprivate
. Double negative; sorry!MAX_BARS
can beprivate
, in that scenario.Thank you.