Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial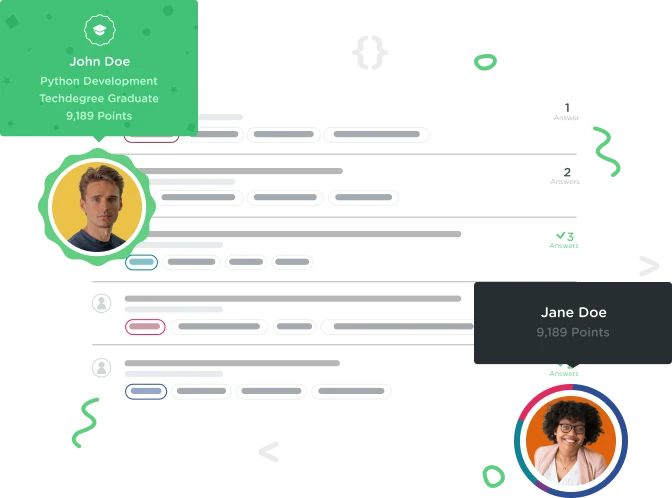
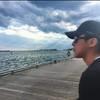
Jinman Kim
5,586 PointsChanging string value
So I am kind of stuck with this question where I need to know how to change the string value. I know string is immutable but I thought it was still able to be reassigned. Last 3 lines were to test if loop is running properly and the length of lists are measured properly. All seems to be good except assigning a new teacher name string. Could anyone help me with this?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
treehouse = {'Kenneth Love': ['Python Basics', 'Python Collections'],
'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
'Jinman Kim': ['Foundation of Java', 'HTML & CSS Basics', 'Introduction to C']}
def num_teachers(treehouse):
num_t = len(treehouse)
return num_t
def num_courses(treehouse):
num_c = 0
for keys in treehouse:
num_c += len(treehouse[keys])
return num_c
def courses(treehouse):
list_c =[]
for keys in treehouse:
list_c += treehouse[keys]
return list_c
def most_courses(treehouse):
max = 0
teacher =""
for keys in treehouse:
if len(treehouse[keys]) > max:
max = len(keys)
teacher = keys
#Below lines are for testing
print(keys)
print(len(treehouse[keys]))
print(teacher)
return teacher
1 Answer

diogorferreira
19,363 PointsHi, to make it easier for yourself I would split both the teacher and courses into different variables,
you can do this with for teacher, courses in dictionary.items():
- Then you can just compare the course length as you were doing.
- I'm not sure if setting the built-in function max is what is causing it to not work? I'd have to test that later maybe.
This is what I came up with hopefully it helps:
def most_courses2(dictionary):
teacher = ''
maximum = 0
for teacher, courses in dictionary.items():
if len(courses) > maximum:
maximum = len(courses)
teacher = teacher
return teacher
Jinman Kim
5,586 PointsJinman Kim
5,586 PointsReally appreciate your help! I'm still a bit confused though as to why splitting the key and value has made a difference.