Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial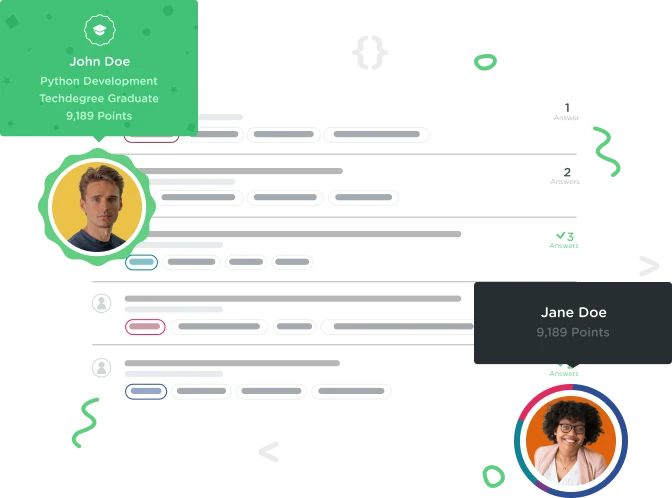

Boipelo Phologolo
4,505 Pointschanging the background color
where am I going wrong? Let's get some practice working with views and colors! In the editor below you have a view controller subclass.
Add a stored property to the view controller named blueColor and assign an instance of UIColor as the default value.
You can use UIColor's RGB initializer method and pass the values 0,0,255,1.0 for red, green, blue and alpha respectively. Alternatively, UIColor has a property that you can use: UIColor.blue.
class ViewController: UIViewController {
let blueColor = UIColor (red:0/255.0, green: 0/255.0, blue: 255/255.0, alpha: 1.0)
let backgroundColor = UIColor.blueColor
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
1 Answer

Nick Kohrn
36,935 PointsEither of the following will work:
let blueColor: UIColor = .blue
let blueColor = UIColor.blue
let blueColor = UIColor(red:0/255.0, green: 0/255.0, blue: 255/255.0, alpha: 1.0)
You are not able to create two properties and make one of them equal to the value of another by implementing them in the way that you have. You can use a computed property, though, to achieve what you're trying to do. The following will allow you to create a blueColor
property and use a computed backgroundColor
property to access the value stored in the blueColor
property:
let blueColor = UIColor.blue
var backgroundColor: UIColor {
return blueColor
}
However, in the instructions for the code challenge that you posted, I don't see where it asks you to create a backgroundColor
property, so I'm not sure if that will cause errors when trying to complete the challenge, but the solution that I provided should work for you.
Thom Benjamin
10,494 PointsThom Benjamin
10,494 PointsIf you're setting blueColor to be the UIColor, you're creating a variable, not a property. So I guess you only have to say:
let backgroundColor = blueColor
I'm not sure about how this works in Swift, but it's worth the try.