Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial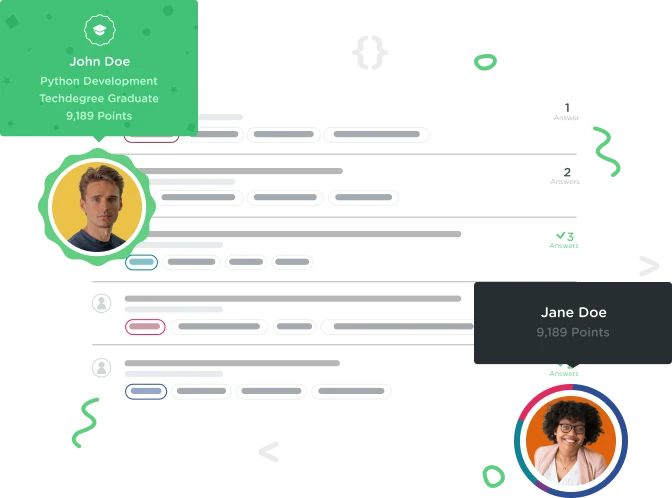

John Balladares
Courses Plus Student 9,828 PointsChanging/toggling Edit Button from "Edit" to "Save"
I tried a few different methods but was unsuccessful
6 Answers
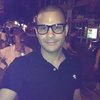
Brian Polonia
25,139 PointsInside of the editTask
function, add a new variable to select the edit button. i.e.
var editBtn = listItem.getElementsByTagName('button')[0];
Then modify your if/else statement to look like the following:
if (containsClass) {
//switch from .editMode
//label text becomes input value.
label.innerText = editInput.value;
editBtn.innerText = "Edit"; //<----- This is what you need to add
} else {
//switch to edit mode
//input value becomes label's text.
editInput.value = label.innerText;
editBtn.innerText = "Save"; //<--------------- Add this also
}
and Inside the index.html look for the <li>
with the class of editMode and change its child <button>
element to have the text of "Save" rather than "Edit".
Also, for the bindTaskEvents
function, I used addEventListener
on its variables rather than onclick
or onchange
events.
Hope this helps.
- Brian
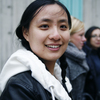
Chen Liu
9,996 PointsI did like this, and it works.
var editButton = listItem.querySelector("button.edit");
if(containsClass) {
label.innerText = editInput.value;
editButton.textContent = "Edit";
} else {
editInput.value = label.innerText;
editButton.textContent = "Save";
}
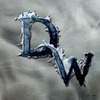
Hugo Paz
15,622 PointsCan you post your attempts here?

Mark Lovett
15,853 PointsBrian, You replaced onclick and onchange with addEventListener ? Or there's more to it than that? What is the syntax you used?
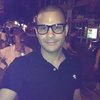
Brian Polonia
25,139 PointsYes. So originally the bindTaskEvents function had onClick and onChange events on its variables. I'll paste the updated code below and point out where I made the change to make use of addEventListener method.
The updated function looks like so:
var bindTaskEvents = function(taskListItem, checkboxEventHandler) {
console.log('bind list items events');
//select taskListItem children
var checkBox = taskListItem.querySelector('input[type=checkbox]');
var editButton = taskListItem.querySelector('button.edit');
var deleteButton = taskListItem.querySelector('button.delete');
//bind editTask to edit button
editButton.addEventListener('click', editTask); //<<----addEventListener here
//bind deleteTask to delete button
deleteButton.addEventListener('click', deleteTask);//<<----------here
//bind checkBoxEventHandler to checkbox
checkBox.addEventListener('change', checkboxEventHandler);//<<----and here
}
Here is a link to this method referenced on the Mozilla Dev Network: Click here
Hope this helps!
- Brian

Mark Lovett
15,853 PointsThanks much. I'll give it a try when I get a chance.

Mark Lovett
15,853 PointsHere is an alternative way to select the edit button:
var editButton = listItem.querySelector(".edit");
By the way, is there any particular reason you decided to change the onclick method to the addEventListener for the buttons?
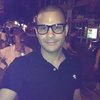
Brian Polonia
25,139 PointsThanks!
And yes. with events handlers such as onclick
or onchange
, consequent events overwrite previous events on the same element. The instructor in this course points it out in the last video of the course titled "Perfect" when he talks about sending an ajax request along with adding a task to the to do list application. So on those events he changed the event handlers to the addEventListener
method because with it an element can listen for multiple events.
Now, in the example I gave above, it would have been totally fine to use the onclick
or onchange
event handlers because we are referencing different elements. But if I can use the addEventListener
method I prefer to do so because its likely that later down the line when you come back to make changes or add features to your application, you may want to listen for more events with some of the same elements and not overwrite any existing event handlers.
Excuse me for the lengthy reply, but hope this clarifies. :)
- Brian
John Balladares
Courses Plus Student 9,828 PointsJohn Balladares
Courses Plus Student 9,828 PointsThanks Brian!
I foolishly tried to create a completely new function dedicated to just changing the innerText of the button. Worked perfectly!
ogechi1
14,455 Pointsogechi1
14,455 PointsHey Brian, Thanks so much! I had also tried to create a new function to no avail... Quick question: is it necessary to change the button element to have the text of "Save"? Does innerText overwrite the existing text? Thanks, Ogechi
Btw, used
var editBtn = listItem.querySelector("button.edit")
to select the button.