Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial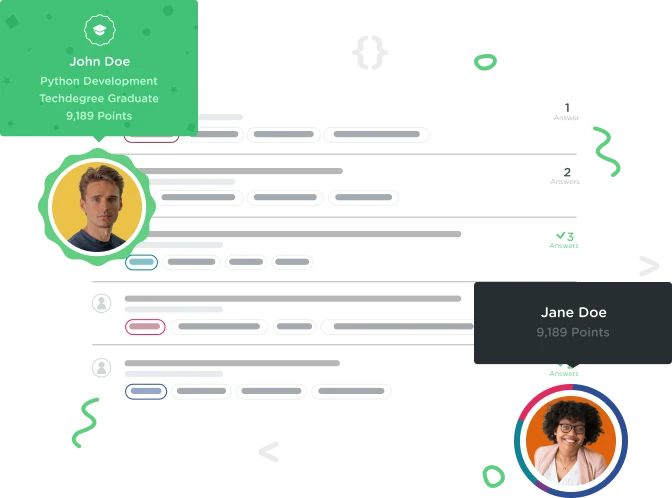

Simen Anthonsen
1,097 PointsCheck if CKRecord array contains a certain record
Iยดve got an CKRecord array consisting of movie objects. How can I, for example, check if the array contains a movie with the title "Jaws" ?
2 Answers
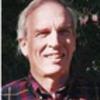
jcorum
71,830 PointsThere are a number of different ways to do this. Here's one that uses a loop inside a function:
//create struct, could also be a class
struct Movie {
var title: String //limited to one stored property, but could easily have many more
}
//create movies
var m1 = Movie(title: "Star Wars")
var m2 = Movie(title: "Pride and Prejudice")
var m3 = Movie(title: "Casablanca")
//create array of movies
let CKRecords = [m1, m2, m3]
//create function to check if array contains a movie
func checkMovie(title: String) -> Bool {
var contains = false
for movie in CKRecords {
if title == movie.title {
contains = true
}
}
return contains
}
//test
checkMovie("Star Wars")
checkMovie("Harry Potter")
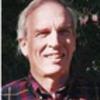
jcorum
71,830 PointsThe contains() method works with Ints, Strings, etc., as expected:
var names = ["B","C"]
names.contains("G") //false
names.contains("B") //true
For arrays of structs, classes (and I don't know what else) contains() requires a different kind of parameter: predicate: (protocol<>) throws -> Bool (i.e., a closure). You can also use the filter() method.
CKRecords.contains({ $0.title == "Casablanca" }) //true
CKRecords.filter( { $0.title == "Casablanca" } ) //true
Simen Anthonsen
1,097 PointsSimen Anthonsen
1,097 PointsCKRecords is of type CKRecord as in CloudKitRecord. What I am doing right now, is what you suggest and loop through the array. But Im wondering if there is a way, that is like this: