Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial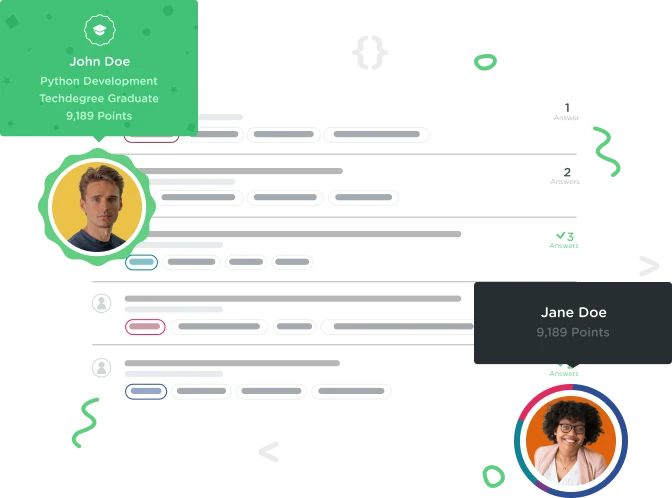
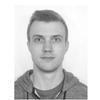
Fredrik Rönnehag
2,342 PointsCheck preceding number in array
I've completed the challenge in two different ways. One with LINQ, which I left in the comment below in RepeatDetector.cs. Is that the correct way with LINQ or can it be done in another way?
Then I did a more basic way using a for loop to check the index with the next index. Is there a way to do it this without a try/catch to avoid the IndexOutOfRangeException? Since I learned it's best to avoid try/catch is possible.
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for(int i = 0; i < sequence.Length; i++)
{
try{
if(sequence[i] == sequence[i + 1]
{
return true;
}
}
catch { }
}
return false;
}
}
}
/*
if(sequence.Length != sequence.Distinct().Count())
{
return true;
}
else
{
return false;
}
*/
1 Answer
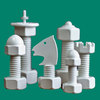
Steven Parker
231,268 PointsJust reduce the upper limit on the loop and you won't cause the exception:
for(int i = 0; i < sequence.Length - 1; i++)
Also, while it might sneak by in the challenge, I don't think the LINQ solution correctly solves the task because it would detect any duplicates. But the stated task is to detect only adjacent duplicates.
Fredrik Rönnehag
2,342 PointsFredrik Rönnehag
2,342 PointsThank you! How would you go by using LINQ to check for the adjacent duplicate?
Steven Parker
231,268 PointsSteven Parker
231,268 PointsGolly, it never occurred to me to do it that way (even when I took this course), but here you go: