Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial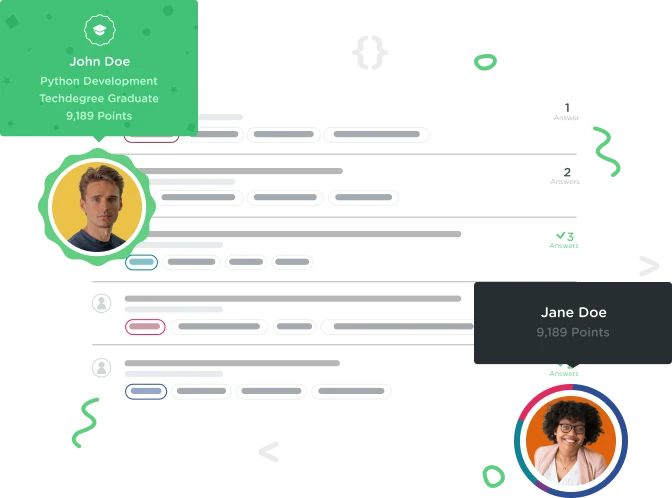

Chris Gauthier
Courses Plus Student 6,434 PointsChecking a counter for negative numbers in the final coding challenge for C# basics
Hello, Im having a problem getting to the inside if statement where I'm checking to see if the input was a negative number. Anybody have any ideas?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var input = Console.ReadLine();
try {
var counter = Convert.ToInt32(input);
var count = 0;
while (count < counter)
{
if (counter <= 0) {
Console.WriteLine("You must enter a positive number.");
}
Console.WriteLine("\"Yay!\"");
count++;
}
}
catch (FormatException) {
Console.WriteLine("You must enter a whole number.");
}
}
}
}
3 Answers

Chris Gauthier
Courses Plus Student 6,434 PointsGreat.... that's solved, thank you. Out of curiosity, what is the facility in c#? That was a very useful feature of perl.
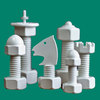
Steven Parker
232,162 PointsCheck the value of counter before you enter the loop.
Since the count will never be less than the counter when the input is negative, the loop code will never run. But this is also something that doesn't belong in the loop because it should only be done one time.

Chris Gauthier
Courses Plus Student 6,434 PointsRight, i did think of that. However, the thing that stumped me is, as i understand it, the program should end once the check for negative numbers expression evaluates to false. In perl, I can do that with and exit command..how do we do this in C#?
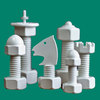
Steven Parker
232,162 PointsThere's a similar facility in C#, but you don't really need it here. Since the negative value will prevent the loop from running, the program just falls through to the end and exits.
Steven Parker
232,162 PointsSteven Parker
232,162 PointsClick on the words "similar facility" in my previous comment, they link to the relevant MSDN page.