Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial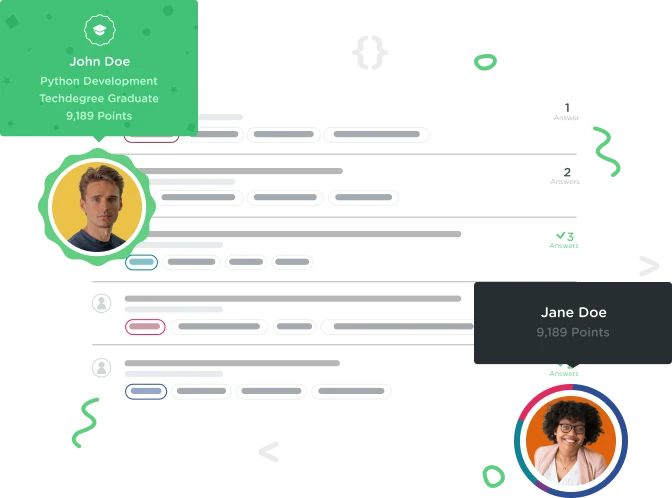

Jaeden Eldred
1,038 PointsChecking a string to verify if it contains only letters and $ sign.
I am attempting to check a String to verify that it only contains letters and the $ sign. I have taken the String and converted it to an array of characters. Then I run the for loop through the array to check to see if it is equal to Character.isLetter(). I keep getting an error when I try to do it this way. If I can get some input or help that would be great! Thanks in advance.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
private String normalizeDiscountCode(String discountCode){
this.discountCode = discountCode;
char [] chars = discountCode.toCharArray();
for (int i = 0; i < chars.length; i++){
if (!Character.isLetter(chars)){
throw new IllegalArgumentException("there are invalid characters in your input.");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer
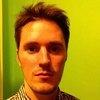
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 Points1) The first line of your normalizeDiscountCode
is: this.discountCode = discountCode;
. But we don't want to do that assignment here, this method is just to normalize the discount code, not to assign it to the property, we're already doing that in the applyDiscountCode
method.
2) You're testing to see if the character is a letter with !Character.isLetter(chars)
, but this is throwing a compiler error (see "Preview" tab) because chars
is an array. You want chars[i]
.
3) Right now you're only checking if it's a letter. But valid characters are letters or the '$' sign, so you need to add that condition.