Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial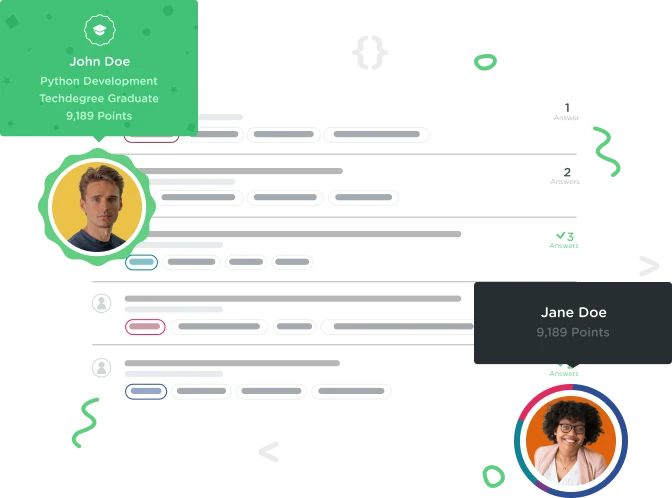
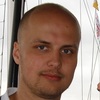
Mariusz Wilk
6,585 PointsChecking if a param is 'undefined'
Hi, so this function doeas NOT check if ar is 'undefined'. Any ideas why? The 'else if' part seems to check for that, but I still get a 'Bummer!'.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
var arrayCounter = function(ar) {
if (ar.isArray) {
return ar.length;
}
if (typeof ar === 'undefined') {
return 0;
}
else {
return 0;
}
}
</script>
</body>
</html>
1 Answer
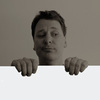
Sean T. Unwin
28,690 PointsYou need to change the syntax for .isArray
.
It should be Array.isArray(ar)
in your example. You can also remove the undefined
clause.
So the full code you could use would be:
var arrayCounter = function(ar) {
if (Array.isArray(ar)) {
return ar.length;
} else {
return 0;
}
}
Reference: Array.isArray()
Mariusz Wilk
6,585 PointsMariusz Wilk
6,585 PointsThx a lot! But how come this method Array.isArray(ar) is so long? Seems inconsistant with the rest of JS methods. Is it some kind of Angular/jQuery/dontKnowWhatElse trick or just a regular JS method?
Sean T. Unwin
28,690 PointsSean T. Unwin
28,690 PointsArray.isArray()
is an inherent method of the Array global object. Other methods, such aspop()
andpush()
are instance methods which inherit from Array.prototype.In essence, when using
Array.isArray()
you are accessing the global object ofArray
itself whereas the 2 other aforementioned methods are accessing methods of Array instances.This is illustrated when creating an Array.