Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial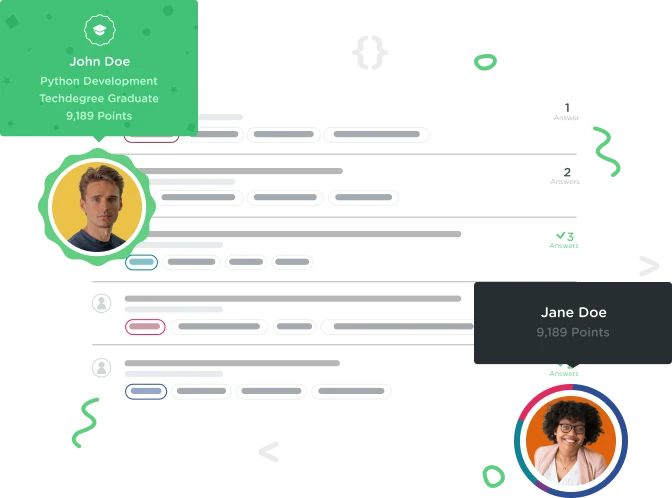

Joel Byrd
507 PointsChecking session variable vs. regular variable
I'm a little confused about the ->with() method flashing data to the session. Isn't data you pass to a template through the ->with() method available in the template by a regular variable reference?
For example, in our TodoListController::show() method, we send the $todo_list variable like so:
return View::make('todos.show')->with('todo_list', $todo_list);
and then in show.blade.php, we access $todo_list just like a regular variable:
<h2>{{{ $todo_list->name }}}</h2>
Similarly, then, in the layout template (main.blade.php), instead of checking the session for the variable "messages", why can't we simply check for the regular variable $messages - since we're passing that variable through:
return Redirect::route('todos.index')->with('message', 'List was created!');
(does it have something to do with the fact that this is in the layout template?)
2 Answers
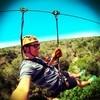
thomascawthorn
22,986 PointsYes, the with() method uses the session to flash information to the view.
You can also use this method
return View::make('blog', array('posts' => $posts));
passing a second parameter (an array) as a second argument to the make() method. Read more.
You should be able to check the $messages variable for any alerts, but I've mostly seen the session checked and this kind of makes sense. Say you have a multiple page form or you're redirected to another page after submission etc.. the $messages variable might not persist, but when stored in the session, these would stay around for any following pages.
This is generally the desired result.. but if it works and you're happy, go for it!

Joel Byrd
507 PointsSo I dug into the source code, and I believe I found the answer - someone correct me if I'm wrong:
The with() method is different depending on which class you are using it with.
For rendering views directly, as in View::make('todos.create')->with(...), this uses \Illuminate\View\View->with(), which simply adds a piece of data to the view:
/**
* Add a piece of data to the view.
*
* @param string|array $key
* @param mixed $value
* @return $this
*/
public function with($key, $value = null)
{
if (is_array($key))
{
$this->data = array_merge($this->data, $key);
}
else
{
$this->data[$key] = $value;
}
return $this;
}
However, when redirecting to a view, as in Redirect::route('todos.index')->with(...), this uses \Illuminate\Http\RedirectResponse->with(), which flashes the data to the session:
/**
* Flash a piece of data to the session.
*
* @param string $key
* @param mixed $value
* @return \Illuminate\Http\RedirectResponse
*/
public function with($key, $value = null)
{
if (is_array($key))
{
foreach ($key as $k => $v) $this->with($k, $v);
}
else
{
$this->session->flash($key, $value);
}
return $this;
}
So, depending on which with() method you are using, you may need to access data directly through a variable, or via the Session.
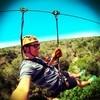
thomascawthorn
22,986 PointsAwesome! Makes sense using the session on a redirect then ;)
Joel Byrd
507 PointsJoel Byrd
507 PointsHmm...then why doesn't the following work (layouts/main.blade.php)?