Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial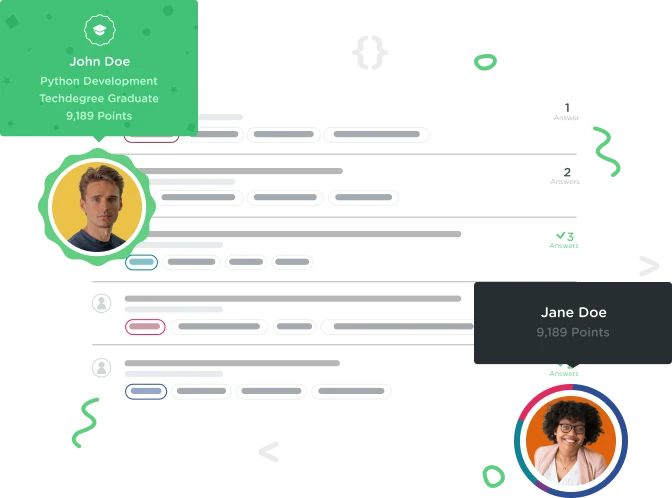
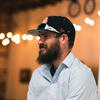
Michael Stedman
Full Stack JavaScript Techdegree Student 13,838 PointsChecking to see if my code meets the standards for the challenge.
My code works 100%, but I was looking to see if anyone else would let me know if they believed I met all the standards for the challenge. I tried to use several things that we have learned over the course so far, so I it has some stuff in there that I don't think was explicitly stated in the instructions. Any and all other comments, suggestions, or critiques would be appreciated.
/* THE CONDITIONAL CHALLENGE INSTRUCTIONS
1. Ask at least five questions.
2. Keep track of the number of questions the user answered correctly.
3. Provide a final message after the quiz letting the user know the number of questions he or she got right.
4. Rank the player. If the player answered all five correctly, give that player the gold crown, 3-4 is a silver crown, 1-2 is a bronze crown, and 0 correct is no crown at all.
*/
/* CHALLENGE QUESTIONS AND ANSWERS
1. Who was the first American president? George Washington
2. Who was the second American president? John Adams
3. Who was the third American president? Thomas Jefferson
4. Who was the fourth American president? James Madison
5. Who was the fifth American president? James Monroe
*/
// SETTING SOME VARIABLES
numberCorrect = 0;
numberIncorrect = 0;
// FIRST QUESTION
var firstPrez = prompt('Who was the first American president?');
if (firstPrez.toUpperCase() === 'GEORGE WASHINGTON' || firstPrez.toUpperCase() === 'WASHINGTON') {
alert('You are correct!');
numberCorrect += 1;
} else {
alert('Sorry, that is incorrect.');
numberIncorrect += 1;
}
// SECOND QUESTION
var secondPrez = prompt('Who was the second American president?');
if (secondPrez.toUpperCase() === 'JOHN ADAMS' || secondPrez.toUpperCase() === 'ADAMS') {
alert('You are correct!');
numberCorrect += 1;
} else {
alert('Sorry, that is incorrect.');
numberIncorrect += 1;
}
// THIRD QUESTION
var thirdPrez = prompt('Who was the third American president?');
if (thirdPrez.toUpperCase() === 'THOMAS JEFFERSON' || thirdPrez.toUpperCase() === 'JEFFERSON') {
alert('You are correct!');
numberCorrect += 1;
} else {
alert('Sorry, that is incorrect.');
numberIncorrect += 1;
}
// FOURTH QUESTION
var fourthPrez = prompt('Who was the fourth American president?');
if (fourthPrez.toUpperCase() === 'JAMES MADISON' || fourthPrez.toUpperCase() === 'MADISON') {
alert('You are correct!');
numberCorrect += 1;
} else {
alert('Sorry, that is incorrect.');
numberIncorrect += 1;
}
// FIFTH QUESTION
var fifthPrez = prompt('Who was the fifth American president?');
if (fifthPrez.toUpperCase() === 'JAMES MONROE' || fifthPrez.toUpperCase() === 'MONROE') {
alert('You are correct!');
numberCorrect += 1;
} else {
alert('Sorry, that is incorrect.');
numberIncorrect += 1;
}
// FINAL RESULT MESSAGE
if (numberCorrect === 5) {
alert('You get a Gold Crown for getting all five answers correct! You had ' + numberIncorrect + ' incorrect.');
} else if (numberCorrect === 4 || numberCorrect === 3) {
alert('You get a Silver Crown for answering ' + numberCorrect + ' correctly. You only had ' + numberIncorrect + ' incorrect.');
} else if (numberCorrect === 2 || numberCorrect === 1) {
alert('You get a Bronze Crown for answering ' + numberCorrect + ' correctly. You had ' + numberIncorrect + ' incorrect.');
} else {
alert('Sorry. You do not get a crown. You answered all five questions incorrectly.');
}
1 Answer
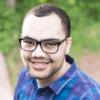
Philip Gales
15,193 PointsThat code looks beautiful! And great use of comments.
If you wanted a nitpicky suggestion, here is mine:
- You can use 'numberIncorrect' in your if statements. This way you can add or remove questions without having to do much work on the if statement.
if (numberIncorrect === 0) {
alert('You get a Gold Crown for getting all' + numberCorrect + ' answers correct! You had ' + numberIncorrect + ' incorrect.');
}
Would always be the best scenario, even if you added more questions.
Michael Stedman
Full Stack JavaScript Techdegree Student 13,838 PointsMichael Stedman
Full Stack JavaScript Techdegree Student 13,838 PointsSweet! Thanks for checking that for me and the suggestion. I was stoke to see on the follow up video (Dave McFarland s solution), that I was pretty darn close to exactly how he did it. Of course, with the minor differences here and there like my use of commenting and him writing the results to the page instead of using an
alert()
. Originally, I had thought to write everything to the page, but I thought that since most of our stuff has been using alert pop ups, that was what he was looking for.After this part of the course, I think I am going to go back and tinker with my code using your "nitpicky" suggestion, just to see how well I can get that to work. Again, thanks.