Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial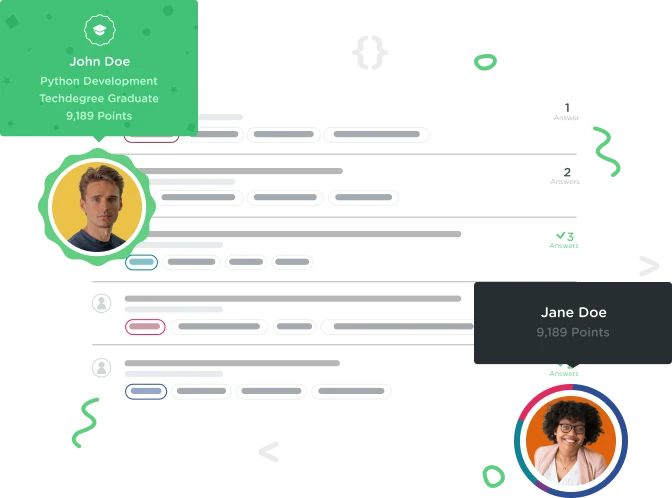

Luke Lee
7,577 PointsChecking Values
var $required = $(".required");
function containsBlanks() {
var blanks = new Array();
$required.each(function(){
blanks.push($(this).val() =="");
});
return blanks.sort().pop();
}
The above codes confused me a lot. The purpose of these codes is collecting value from each required field, and push them into an Array, then check if any of them are empty. Am I right?
Then why do we make the array null?
blanks.push($(this).val() =="");
2 Answers

Nick Fuller
9,027 PointsHi Luke!
I just want to confirm I have the correct code, so please review it.
var $required = $(".required");
function containsBlanks() {
var blanks = new Array();
$required.each(function() {
blanks.push($(this).val() === "");
});
blanks.sort().pop();
}
If so, you're correct about the logic of the code!
But we aren't making the array null. Remember the assignment operators vs. the equality operators. One = sign is assignment. 3 === equal signs is equality, which means "Is the value on the left the same as the value on the right?"
For instance, my name is Nick and if I store it in a variable as a string and compare it to yours are they the same?
var nick = "Nick";
var luke = "Luke";
alert(nick === luke);
This will return false, because obviously our names aren't the same.
So looking again at this logic
$(this).val() === "";
We can see that we are checking if the value of the current item in the iteration, represented by the jQuery keyword $(this), is the same as an empty string "" which will either be true or false.
We then push that result into the array called blanks.
blanks.push($(this).val() === "");
Finally when we are done iterating, we sort our new array of "blanks" by its values. Doing so will move any instances of the value true to the end of the array. Then we use the pop() function, which pops off the last value of the array and we return that. So if the array is full of falses ([false, false, false, false]) then the pop function will grab the last false and return it. But, if the array has even just one true value ([false, true, false, false]) it will be re-sorted to [false,false,false,true] and we will pop the last value, true, and return it!
SO long story short... This function iterates over the $required collection, checking each elements' value to see if any are blank (an empty string ""). If any of them are, the function will return true, if not the function will return false.

Luke Lee
7,577 PointsAh, I see, I was confused about ===. Thank you for your help!