Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial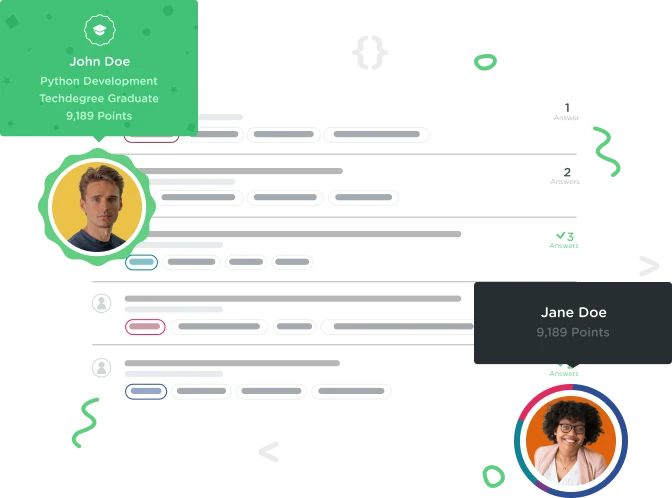

Ediju Rodrigues
5,214 Pointscheck_speed
Define a method named check_speed that takes a single number representing a car's speed as a parameter. If the speed is over 55, check_speed should print the message "too fast". If the speed is under 55, check_speed should print the message "too slow". And if the speed is exactly 55, check_speed should print the message "speed OK".
Man Ruby is not for me! Whats the correct way to do this challenge?
def check_speed(kmh)
end
check_speed(55)
if check_speed > 55
puts "to fast"
end
if check_speed < 55
puts "to slow"
end
if check_speed == 55
puts "speed OK"
end
3 Answers

Luke Pettway
16,593 PointsYou have a few things going on, the first thing that is probably the least obvious is that your puts strings need to spell the word too
and not to
. I'm guessing that the way they test your code looks for the correct spelling.
puts "to slow"
# should really be
puts "too slow"
The next issue is that your control structure of if statements is not inside of your method declaration, so you should move them inside:
def check_speed(kmh)
if check_speed > 55
puts "to fast"
end
if check_speed < 55
puts "to slow"
end
if check_speed == 55
puts "speed OK"
end
end
Finally the last issue is that you are using the method name check_speed
where you should be using the name of the method parameter (kmh), which just for posterity you should change to speed, and replace each instance of check_speed
with it:
def check_speed(speed)
if speed > 55
puts "too fast"
end
if speed < 55
puts "too slow"
end
if speed === 55
puts "speed OK"
end
end
Let me know if the helps you progress and if you have any other questions.

Ediju Rodrigues
5,214 PointsLuke Pettway, Thank you very much for explaining my mistakes! Maybe Im making silly questions here, but I'm kinda confused! I don't understant, thru out the course they always atribute a value to the parameter and use it to make the comparisons, but in here for example the "speed" parameter doesn't have a value how i can know when its bigger ,less or equal to 55? What i'm missing here?

Luke Pettway
16,593 PointsThe value of speed is what is passed in as the single argument to the method
check_speed(55) <--- 55 is the value of speed check_speed(55 = speed) is pretty much what is going on here j
speed
itself is just a placeholder for the value you pass in, sometimes you have more than one value, for example you might have a method that calculates the speed of something which you would need distance and time and thus would look like:
def calculate_speed(distance, time)
...
end
The value of distance and time are equal to the arguments when the method is called
calculate_speed(55, 100) distance is 55 and time is 100.

Ediju Rodrigues
5,214 PointsAlicja Dul, thank you very much for helping me \o/

Ediju Rodrigues
5,214 PointsLuke Pettway thank you ;)
Alicja Dul
8,589 PointsAlicja Dul
8,589 PointsHi,
This works for me:
def check_speed(speed) if speed < 55 puts "too slow" end if speed > 55 puts "too fast" end if speed == 55 puts "speed OK" end end
Regards