Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial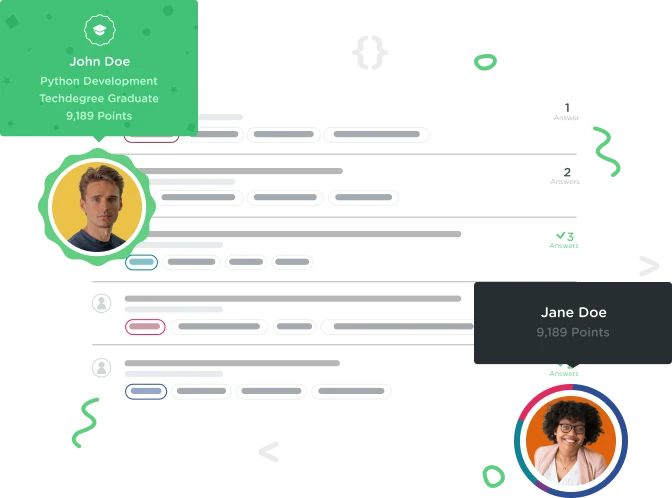
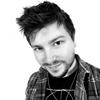
Derek Miller
6,466 PointsChoose your own adventure
Hey guys! I'm trying to build a 'Choose your own adventure' app using javascript, html, and just a little css. I have been able to create the first question, but once they make their decision, I don't know how to make that first bit of the story disappear and then queue in the next part of story text. Is there a bit of js code that makes text disappear once a button is clicked, and is replaced with whole new code? Does this make sense?
Thanks!
2 Answers
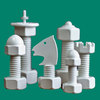
Steven Parker
230,688 PointsSure, and there's a number of ways to do this, but here's one simple one. Enclose each bit in a container element, like a <div>
, and give it a unique ID name. Then, in your CSS set the "display" property to "none" on all the ones that should not be visible to start with. For example, if five containers had ID names of "q1" to "q5", you might have this in your CSS:
#q2, #q3, #q4, #q5 { display: none; }
Then, in the button click handler, you might have this code to make q1
vanish and q2
appear:
document.getElementById("q1").style.display = "none";
document.getElementById("q2").style.display = "block";
If you continue with the JavaScript courses here, you will learn this and other was of performing dynamic presentation changes on your page.
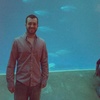
Kyle Johnson
33,528 PointsYou can do this by adding a style to the part of the story that you want to disappear. The CSS is simple. Just create a class with display: none;
. Then you want to add an addEventListener
to the button and put the callback function that will apply the CSS class to that part of the story. Below is a very simple example.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<!-- CSS -->
<style>
.hidden {
display: none;
}
</style>
</head>
<body>
<p id="hideThis">Make this disappear!</p> <!-- What to hide -->
<button id="btn1">Click Me!</button> <!-- Button to trigger hide -->
<!-- Javascript -->
<script type="text/javascript">
const p = document.getElementById('hideThis');
const btn = document.getElementById('btn1');
<!-- Add hidden css style to hideThis ID -->
function hideClass() {
hideThis.className = 'hidden';
}
btn.addEventListener('click', hideClass); <!-- Execute hideClass on click -->
</script>
</body>
</html>