Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial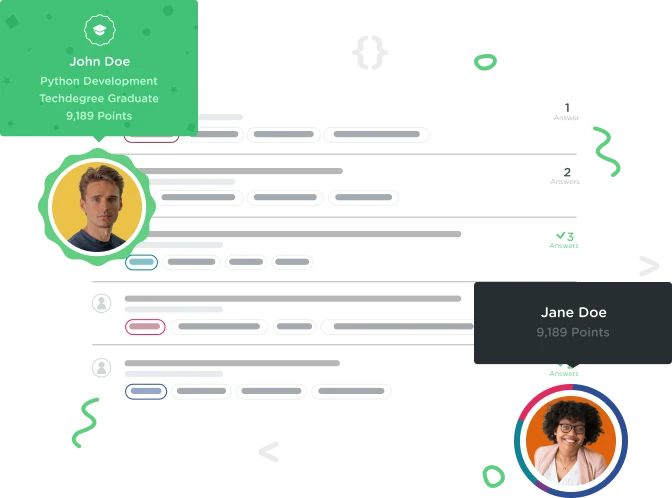

Adam Del Conte
7,250 PointsClarifying the {name} and {food} for this ding dang Panda ;p
Y'all, please tell me what I'm doing wrong for step 2 of this problem.
I cannot see the problem.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
name = 'Bao Bao'
def __init__(self, name, age):
self.name = name
self.age = age
self.is_hungry = True
def eat(self):
self.is_hungry = False
self.name = name
self.food = food
return '{} eats {}.'.format(self.name, self.food)
2 Answers
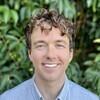
Asher Orr
Python Development Techdegree Graduate 9,408 PointsHi Adam! The problem is within your "eat" method.
These 2 lines specifically:
self.name = name
self.food = food
Let me show you why:
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
#the above line says: for all instances of the Panda class, the food attribute is 'bamboo'
name = 'Bao Bao'
#note: you can delete this line (name= 'Bao Bao'). I'll get into why later.
def __init__(self, name, age):
self.is_hungry = True
self.name = name
#the above line sets the "name" attribute on any instance of your Panda class to whatever you set it to.
self.age = age
def eat(self):
self.is_hungry = False
return '{} eats {}.'.format(self.name, self.food)
When you click "Check Work," the checker for this Treehouse challenge is running code like this:
attempt = Panda('Bao Bao', 7)
#this creates the instance of the class.
#the __init__ method wants arguments for the name and age attributes, so they get added here.
attempt.eat()
#now it runs the 'eat' method on this instance of your Panda class, which it named attempt.
Running attempt.eat() will return "Bao Bao eats bamboo."
'Bao Bao' is the name, since the checker passed in the argument 'Bao Bao' for the name attribute. (this is why you can delete line 4.) The name is attribute is supposed to be set when you create an instance- that's why the init method takes name as an argument.
'Bamboo' is the food attribute, because 'bamboo' is the food attribute for ANY instance of the class.
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
Your eat method is getting the information about its attributes (self.name, self.age, self.species, etc) from 2 places:
A) The Class attributes
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
If an attribute should always be the same, you make it a Class attribute.
B) The dunder init method.
def __init__(self, name, age):
These are for attributes that might change, depending on the instance. For example:
bao_bao = Panda('Bao Bao', 7)
basi = Panda("Basi", 2)
bobo = Panda("Bobo", 5)
Now, to loop back to your original code:
def eat(self):
self.is_hungry = False
self.name = name
#Python goes "what?" self.name was defined in the initializer. Now you want it to equal a variable called "name"
#I don't know what that variable is, so I'm hitting you with a NameError.
self.food = food
#Python again goes "what?" self.name is always 'bamboo'. Now you want it to equal a variable called "food"
#I don't know what that variable is, either.
return '{} eats {}.'.format(self.name, self.food)
If you remove those 2 lines, your code should pass the challenge. I hope this helps- let me know if you have any questions!
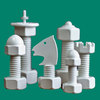
Steven Parker
230,274 PointsNeither name nor food are defined inside the method "eat". But they don't need to be, since they are both defined when the instance is created, and can be referenced via "self.".

Adam Del Conte
7,250 PointsThank you, Steven.
Adam Del Conte
7,250 PointsAdam Del Conte
7,250 PointsThank you so much! Cleared things up perfectly. Now I just have to get through Step 3. Might be back soon with a follow up question 😄