Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial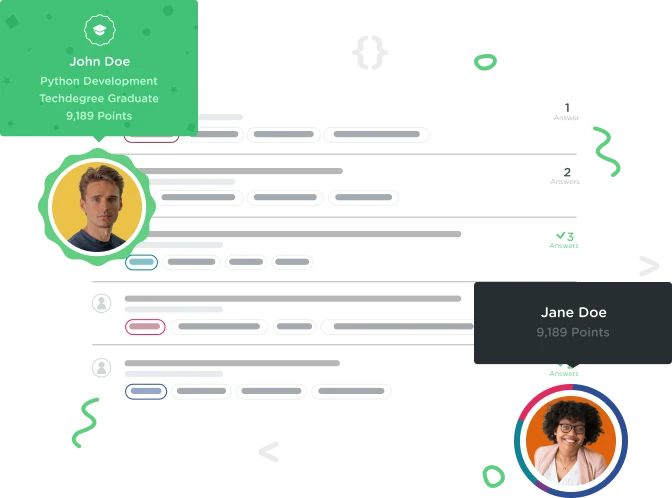

Matt Conway
1,572 PointsClass
Not sure where to put "name" per the challenge instructions ...
Challenge Task 1 of 1
In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.
/Users/mattconway/Desktop/Screen Shot 2016-01-24 at 2.50.20 PM.png
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
var name: String
var location: Location
init(latitude: Double, longitude: Double) {
self.name = name: String
self.location = location(latitude: Double, longitude: Double)
}
}
let someBusiness = Business(name: String, latitude: Double, longitude, Double)
4 Answers
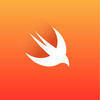
Steven Deutsch
21,046 PointsHey Matt Conway,
You have the properties in your Business class set up correctly. We have to make some changes to the initializer though.
Your initializer needs to take two parameters that match the type of your classes properties. Therefore, you need to pass in one String parameter and one Location parameter. You then refer to the properties of your class by using self, and assign the values of these parameters to them.
Next, when we create an instance of the Business class, we have to pass in what the initializer requires. You will have to pass in a String as the first argument, and an instance of Location as the second.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "Steven", location: Location(latitude: 20.0, longitude: 50.0))
Hope this helps! Good Luck!

Matt Conway
1,572 PointsMakes sense, but strange it doesn't work on neither playground nor challenge. It says there is a missing comma at the end of the code. I can't really see your end after 50.0). Here is what I wrote, what is wrong?
struct Location { let latitude: Double let longitude: Double } class Business { let name: String let location: Location init(name: String, location: Location) { self.name = name self.location = location } } let someBusiness = Business(name: "Matt", location: Location(latitude: 20.0, longitude: 50.0)

Matt Conway
1,572 PointsNever mind, I got it. I forgot to close it with the extra parenthesis at the end.

Dale Bailey
20,269 PointsHi I think that this answer is a little cleaner than Steve's and a closer to what they wanted. Instead of passing the location into the instance of Business (someBusiness) you pass the requirements of the init which is name, latitude and longitude, inside the init you set "self.location" to an instance of the "location" struct passing in the latitude and longitude properties you set on "SomeBusiness" This way you can see that the init is referencing a struct
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, latitude: Double, longitude: Double) {
self.name = name
self.location = Location(latitude: latitude, longitude: longitude)
}
}
let someBusiness = Business(name: "Flowmoco", latitude: 50.41549699999999, longitude: -5.073718999999983)
Here is another way of looking at it Treehouse won't accept this but it is valid and may make more sense too some
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
let latitude: Double // optional
let longitude: Double //optional
init(name: String, latitude: Double, longitude: Double) {
self.name = name
self.latitude = latitude
self.longitude = longitude
self.location = Location(latitude: latitude, longitude: longitude)
}
}
let someBusiness = Business(name: "Flowmoco", latitude: 50.41549699999999, longitude: -5.073718999999983)
someBusiness.latitude // 50.41549699999999
someBusiness.longitude // -5.073718999999983
someBusiness.name // Flowmoco
someBusiness.location // Location
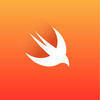
Steven Deutsch
21,046 PointsDale Bailey, What happens when you already have an instance of Location? Forgive me if I'm wrong, but - Your initializer now requires that you pass in a latitude and longitude every time, regardless of whether you already have a Location instance. With my code, you can either pass in an instance of Location directly or create one within the initializer. Also, why have a data structure for Location in the first place if you're going to have them repeated as properties in your Business class?
Thanks, Steve