Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial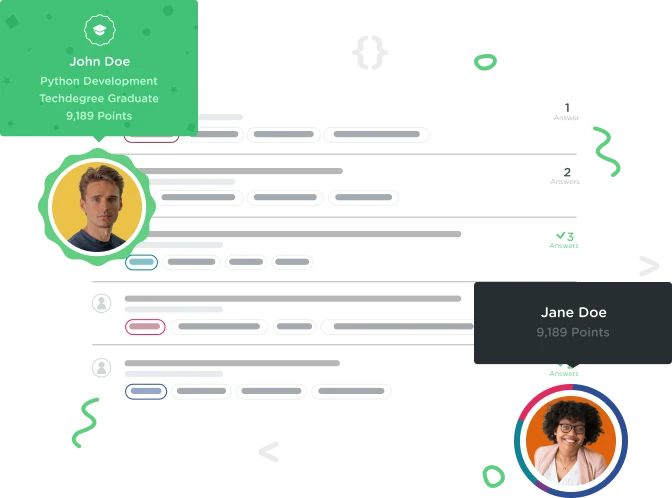

Amber Tai
596 PointsClass BankAccount example
Hello there,
Could you help me understand the following?
I don't understand the connect from class to bank_account = BankAccount.new("XX XXX") I understand the whole class coding process but how we we know to code like that afterwards?
What is the different in here between "print" and "puts"? print is to 'copy' what we type in while puts will do the function like math? Just want to know if I understand it correctly. def deposit print "How much would you like to deposit? " amount = gets.chomp @balance += amount.to_f puts "$#{amount} is deposited."
Why not put @amount like @balance? @blance is a variable but amount seems to be a variable too depending how much it gets from the string. How will we know when to put @?
Thanks!!! : )
6 Answers
William Li
Courses Plus Student 26,868 PointsYou ask some pretty good questions here, I'll try to answer them one at a time.
difference between puts & print
Simply say, the only difference is that puts always insert a newline at the end of the print String, whereas print doesn't start a new line.
puts "hello"
puts "hello"
puts "hello"
# => hello
# => hello
# => hello
print "hello"
print "hello"
print "hello"
# => hellohellohello
That's pretty much all you need to know about puts & print.
local variable VS Instance variable.
Why not put @amount like @balance? @blance is a variable but amount seems to be a variable too depending how much it gets from the string. How will we know when to put @?
I think you're referring to this code block, right?
def deposit
print "How much would you like to deposit? "
amount = get.chomp
@balance += amount.to_f
puts "$#{amount} deposited."
end
- @balance here is instance variable, You can think of instance variable as property associated with the BankAccount object. Later when you get into topic Accessor, you can see that the value of instance variable of an object can easily be retrieved via dot notation.
- amount here is local variable, it existed only within the scope of the deposit function, you may think of local variable as helper to store temperate value, every time you call the deposit function, a new amount local variable was created, and as soon as function execution is finished, this variable is erased from the memory.
Now to answer your question, yes, you can totally make amount instance variable, just initialize it on the initialize method, then you can start using @amount, and the code would work pretty much the same.
However, that's not a very good Object Oriented design. Remember Instance Variable is a property of an object, @balance is instance variable because every Bank account object should and must have a balance property, that make sense; but does it make sense to make amount one as well?
Let's take another look at what the amount variable actually does in the context of the deposit function. amount local variable stores the value according to user input, then its value was added to the @balance, then puts print out how much was deposited.
Now, by looking at what purpose amount serves, I say that Jason's choice to make it local only to the deposit function is sound, because it pretty much only existed to help deposit function to do its job; If you were to make it instance variable @amount instead, it'd become a property of the Bank account object. Keep in mind that you should make something instance variables only if they are essential properties which must be associated with an object, in another word, some value the object must always keep track of. I don't think it makes much sense to keep an instance variable storing how much the amount was last deposited.
Hope you find it helpful, yeah, many of the OOP concepts can take time to fully understand., let me know if you have further question.
William Li
Courses Plus Student 26,868 PointsIt's interesting that the coding camp that I am planning to attend to sent me some courses to read before it starts.
Congrats on attending Code camp. Which one are you going to? Just my curiosity.
Now I got several people saying that the some courses that I have been watching is gonna retired.
Yeah, the software development world is evolving fast, it's nice that here at Treehouse, new materials are constantly being produced to keep things up-to-date with the development landscape; and I'd suggest you give feedback to your Code Camp staffs to make sure the resource handouts they're handing over to students are updated as well.
In the other words, outdated?
Ummm ... I can't say it's outdated, the Ruby Foundations course was recorded using Ruby Version 1.9.x, though I'd say 95% of the course materials are applicable to the current version of Ruby; however, since Treehouse has produced a series of Ruby courses as replacement for Ruby Foundation using version 2.x, and it make use of Treehouse's new Workspace as coding platform, it's better that you learn from the new courses.
What's following after the bank_account = BankAccount.new("") bank_account.class # => BankAccount
This line
bank_account.class # => BankAccount
the .class is a method, much like deposit and show_balance defined in the BankAccount class definition; they are all methods.
What .class does is to tell you what class the bank_account object belongs to; it returns BankAccount
, make sense, right?
You may say, wait a minute. My BankAccount class had deposit and show_balance methods defined, but I have never defined a class method, where does it comes from? It's a predefined method in Ruby, and since Ruby is the purest Object Oriented language, everything in Ruby is an object, you can try using the .class method call on pretty much anything.
[1,2,3].class #=> Array
"abc".class #=> String
3.class #=> Fixnum
Do not confuse the .class with the class keyword.
class BankAccount # class here is a keyword
# detail omitted
end
When you use class by itself, it's a keyword, it triggers the class definition; whereas in bank_account.class, the .class is a method call. They are 2 totally different things. The key thing to take note here is that, whenever you see something in the form of object.xxx, that's the dot notation, it's used for calling a method on an object.

Amber Tai
596 PointsHi William,
Thanks for the detailed reply. Will try to absorb with time. The camp that I am thinking about joining is located closer to where I live. It's local in a sense. Here is the web: https://www.alphacamp.co/sg/ Thought it's link is sg but I am in Taipei, Taiwan. : )
William Li
Courses Plus Student 26,868 PointsCool, it looks promising too . Hope you have a great time there. And let me know if you have further questions.
William Li
Courses Plus Student 26,868 PointsOften I have to read it several times. I wonder if it's the path that every developer experiences before. : )
Yes, it's totally normal, it can be quite intimidating at first.
class BankAccount
def initialize(name)
@transactions = []
@balance = 0
end
def deposit
print "How much would you like to deposit? "
amount = get.chomp
@balance += amount.to_f
puts "$#{amount} deposited."
end
def show_balance
puts "Your balance is #{@balance}."
end
end
bank_account = BankAccount.new("Jason Seifer")
Also, on my first paragraph, I don't quite understand the bank_account = BankAccount.new("") and the rest. I mean after I write the class BankAccount, how will I know by using the bank_account 'format' the and rest to create things and make it function?
Okay, you have trouble understanding this line bank_account = BankAccount.new("Jason Seifer")
?
You've previously defined a BankAccount class here.
class BankAccount
# detail omitted
end
Here's a key point to understand -- class definition by itself isn't doing anything interesting, it only serves as blueprint (or specification) for constructing new BankAccount object.
To put it in plain English, think of class definition in OOP as cookie cutter, and object as cookie. Cookie cutter defines the shapes and size of the cookie, it's a tool you use to cut out cookies from dough.
That's exactly what this line does bank_account = BankAccount.new("Jason Seifer")
.
- In Ruby, .new() is how you create a new object based on class definition
-
BankAccount.new("XXXXX") creates a new BankAccount object based on the class definition of BankAccount, and then assign it to the variable bank_account; it takes one argument -- "XXXXX", why? because in the class definition body, the
def initialize(name)
requires a name argument.
I hope that I am able to help you understand these OOP concepts a little bit.
Right now you're at a pretty early stage of learning Ruby OOP, it's okay that you don't get all these terminology and concepts at the first try, it's online course that you can re-watch as many times as needed; but it's important that you go on with the course and learn more about OOP thinking, because they help you see the bigger picture.
One last thing, this Ruby Foundation course is very much retired. You should switch to a more updated version of this course.

Amber Tai
596 PointsThanks William! Your explanation does help me a lot. I will watch the two videos.
It's interesting that the coding camp that I am planning to attend to sent me some courses to read before it starts. Now I got several people saying that the some courses that I have been watching is gonna retired. In the other words, outdated? How will I know if the camp is really helping me out? (off the track I know sorry. You don't need to answer this.) : P
I have one more question. What's following after the bank_account = BankAccount.new("") bank_account.class # => BankAccount or in the extra credit part, the example of your_name.name_length, that is the logic flow to become this way?
Thank you very much for these!
Ann
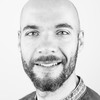
Alberto Ferioli
15,672 PointsThanks a lot William, all of these was really helpful also for me!
Amber Tai
596 PointsAmber Tai
596 PointsHi William,
Thanks the detailed explanation. I understand better of the concepts now. Often I have to read it several times. I wonder if it's the path that every developer experiences before. : )
Also, on my first paragraph, I don't quite understand the bank_account = BankAccount.new("") and the rest. I mean after I write the class BankAccount, how will I know by using the bank_account 'format' the and rest to create things and make it function? Not sure how to describe that. But let me know if you get a gist of it. Thanks!