Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial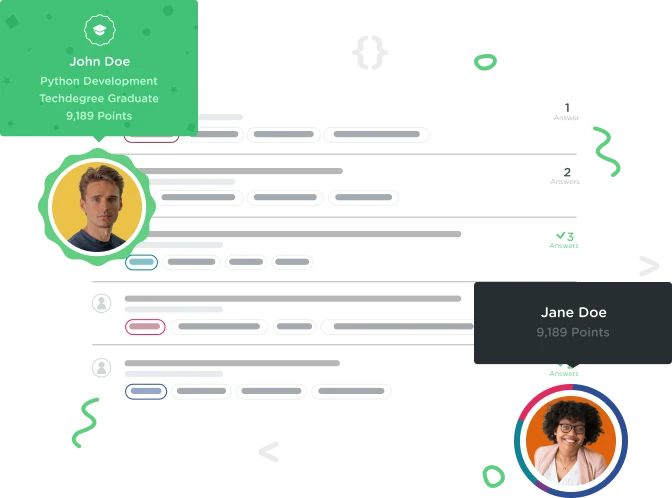

omri golan
Courses Plus Student 15,836 Pointsclass challange
this is the challange i dont understand what am doing wrong can you help me?
Add a score method to Game that takes a player argument. The player argument will be either 1 or 2. Increase that player's value in self.current_score by 1. You'll need to adjust the index (i.e. player = 1 means self.current_score[0] needs to increase).
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self,player):
if player == 1:
return self.current_score[0] += 1
else:
return self.current_score[1] += 2
thanks in advance
[MOD: added ```python markdown formatting-cf]
4 Answers
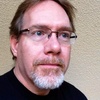
Chris Freeman
Treehouse Moderator 68,423 PointsThere are two issues. The task asks only to update the values not return them and the score increment for player two should also be 1:
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(self,player):
if player == 1:
self.current_score[0] += 1 # <-- removed return
else:
self.current_score[1] += 1 # <-- changed to 1, removed return
Also, avoid mixing tabs and spaces.

Richard Nesbitt
6,775 PointsI completed this challenge in a simpler fashion:
def score(self, player):
self.current_score[player - 1] += 1 #makes the index out of the player number

Matt Nickele
468 Pointsclass Player:
def __init__(self):
self.current_score = [0, 0]
print (self.current_score)
def score(self,player):
if player == 1:
self.current_score[0] = 1
return self.current_score
else:
self.current_score[1] =+ 2
return self.current_score
def __str__(self):
#This is a print function
return str(self.current_score)
a = Player() b = Player.score(a,1) print (a)
Here is what I did. You need a print statement str so you can print your created object and not just the python name for it that means nothing. I personally like to make chances before returning but that is a choice. I hope this helps

omri golan
Courses Plus Student 15,836 Pointsthank you very much for the help :)
Evan Colvin
1,190 PointsEvan Colvin
1,190 PointsThanks! That helped a lot!
Quick question: without a return statement, how does the score function actually do anything?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThe
score
function is bound to an instance ofGame()
. Theself
argument represents the specific instance. Since it operates onself.current_score
it is changing a particular instance attributes and doesn't need to return anything.These bound functions are called methods. Methods can return objects.
score()
does not. The default return if not stated isreturn None