Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial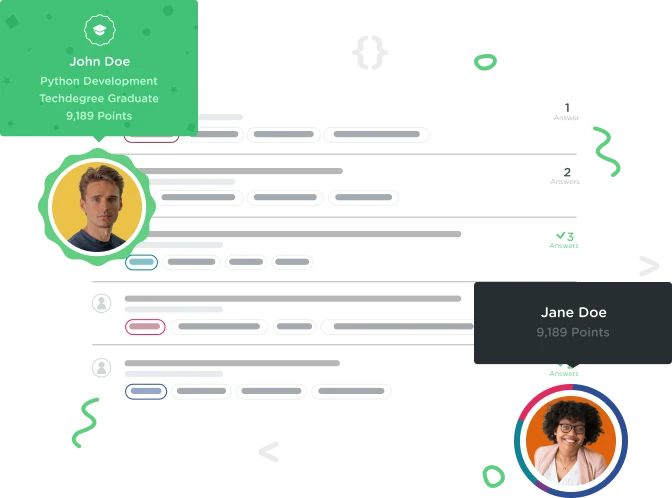

Matthew Brown
1,652 PointsClass Challenge Help
I apparently do not understand initializers......I do not understand what I have to do at all
2 Answers
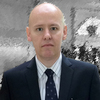
Nathan Tallack
22,159 PointsYeah, they are a little tricky. Especially when sometimes you don't have to write them because they happen automagically (like they do with struct types).
Think of it like this.
The property of a type (that is an immutable value (defined with let) or a mutable value (defined with var)) needs to be set with a value when you first create an object of that class type (unless we consider optionals, but lets ignore that for now).
Consider the following Class type and my use of it. It is a pointless task without methods, but focus on the property.
class Bucket {
var contents: String
}
So my Bucket class can allow me to create objects that have a property of contents. But without an initializer method to tell my object how to set that variable I can't use it.
So, I make the initializer to tell it how to set that variable.
class Bucket {
var contents: String
init(contents: String) {
self.contents = contents
}
}
So now, that init method (remember, a method is a function inside a class) is used each time I try to create an object of type Bucket. Xcode will prompt me to pass in an parameter of type String named contents. And that init function will assign it to that property (remember, that var that i declare at the start of my class) so that it is configured.
let waterBucket = Bucket(contents: "water") // This creates the object
waterBucket.contents // This prints out the string "water"
That ability to access the objects property uses another method called a "getter" which is automagically created for your class by the compiler. But like optionals, that is another lesson for another time. ;)

Matthew Brown
1,652 PointsThank you so much! I appreciate your help. That helps me out a lot.