Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial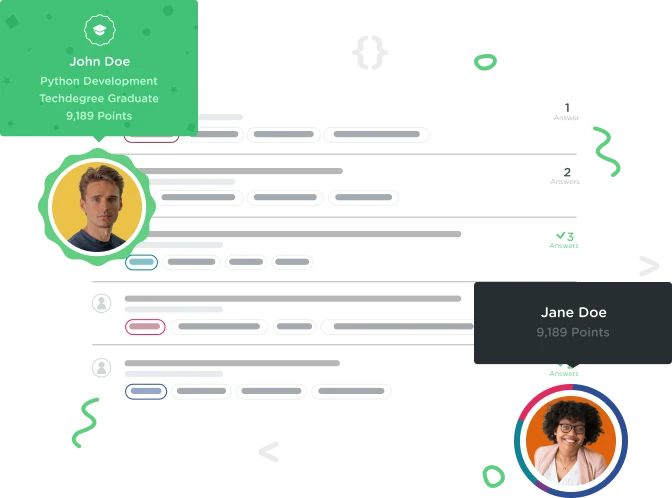
Ryan Doyle
8,587 PointsClass Inheritance Stuggles
Ok, I am in this challenge, and I am totally stuck.
I do get the idea (kind of) of overriding the init in a subclass to initialize an instance of the subclass using the superclass, but I am really struggling in understanding when to use the "self." and when to use "super.". I think that might be what I messed up in the code, but even if by some random chance I got those right an it's something else, it would be helpful if someone could give a dummies version of what the difference is between using self. and parent. when using the subclasses...
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
self.getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Doc", lastName: "Person")
someDoctor.getFullName()
1 Answer

Alexander Smith
10,476 PointsYou've pretty much got it. Instead of self.getFullName(), override it and you're good.
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: firstName, lastName: lastName)
}
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Doc", lastName: "Person")
someDoctor.getFullName()
sugabelly
2,524 Pointssugabelly
2,524 PointsThis confuses me too. Why do you need to override the init if the only thing you're changing in the subclass is what is returned by the function?
Why can't you just have override func without needing to have override init beforehand as well?