Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial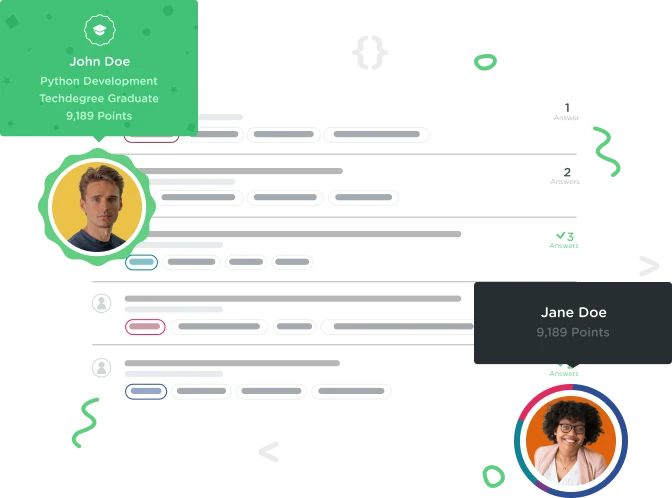
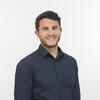
Isaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsClass isn't being applied to crown svg
I'm having trouble understanding why the 'is-high-score' class isn't being assigned to my svg elements? Everything in React dev tools is showing that the highScore functions are working correctly...
Here is my code for the three files:
// Icon.js
import React from "react";
import PropTypes from "prop-types";
const Icon = (props) => {
return (
<svg
viewBox="0 0 44 35"
className={props.isHighScore ? "is-high-score" : null}
>
<path
d="M26.7616 10.6207L21.8192 0L16.9973 10.5603C15.3699 14.1207 10.9096 15.2672 7.77534 12.9741L0 7.24138L6.56986 28.8448H37.0685L43.5781 7.72414L35.7425 13.0948C32.6685 15.2672 28.3288 14.0603 26.7616 10.6207Z"
transform="translate(0 0.301727)"
/>
<rect
width="30.4986"
height="3.07759"
transform="translate(6.56987 31.5603)"
/>
</svg>
);
};
Icon.propTypes = {
isHighScore: PropTypes.bool,
};
export default Icon;
// Player.js
import React, { PureComponent } from "react";
import PropTypes from "prop-types";
import Counter from "./Counter";
import Icon from "./Icon";
class Player extends PureComponent {
static propTypes = {
changeScore: PropTypes.func.isRequired,
removePlayer: PropTypes.func.isRequired,
isHighScore: PropTypes.bool,
name: PropTypes.string.isRequired,
score: PropTypes.number.isRequired,
id: PropTypes.number.isRequired,
index: PropTypes.number.isRequired,
};
render() {
const { name, id, score, index, removePlayer, changeScore } = this.props;
return (
<div className="player">
<span className="player-name">
<button className="remove-player" onClick={() => removePlayer(id)}>
✖
</button>
<Icon isHighScore={this.props.isHighScore} />
{name}
</span>
<Counter score={score} index={index} changeScore={changeScore} />
</div>
);
}
}
export default Player;
// App.js
import React, { Component } from "react";
import Header from "./Header";
import Player from "./Player";
import AddPlayerForm from "./AddPlayerForm";
class App extends Component {
state = {
players: [
{
name: "Guil",
score: 0,
id: 1,
},
{
name: "Treasure",
score: 0,
id: 2,
},
{
name: "Ashley",
score: 0,
id: 3,
},
{
name: "James",
score: 0,
id: 4,
},
],
};
// player id counter
prevPlayerId = 4;
handleScoreChange = (index, delta) => {
this.setState((prevState) => {
// New 'players' array – a copy of the previous `players` state
const updatedPlayers = [...prevState.players];
// A copy of the player object we're targeting
const updatedPlayer = { ...updatedPlayers[index] };
// Update the target player's score
updatedPlayer.score += delta;
// Update the 'players' array with the target player's latest score
updatedPlayers[index] = updatedPlayer;
// Update the `players` state without mutating the original state
return {
players: updatedPlayers,
};
});
};
getHighScore = () => {
const scores = this.state.players.map((p) => p.score);
const highScore = Math.max(...scores);
if (highScore) {
return highScore;
}
return null;
};
handleAddPlayer = (name) => {
this.setState((prevState) => {
return {
players: [
...prevState.players,
{
name,
score: 0,
id: (this.prevPlayerId += 1),
},
],
};
});
};
handleRemovePlayer = (id) => {
this.setState((prevState) => {
return {
players: prevState.players.filter((p) => p.id !== id),
};
});
};
render() {
const highScore = this.getHighScore();
return (
<div className="scoreboard">
<Header players={this.state.players} />
{/* Players list */}
{this.state.players.map((player, index) => (
<Player
name={player.name}
score={player.score}
id={player.id}
key={player.id.toString()}
index={index}
changeScore={this.handleScoreChange}
removePlayer={this.handleRemovePlayer}
ishighScore={highScore === player.score}
/>
))}
<AddPlayerForm addPlayer={this.handleAddPlayer} />
</div>
);
}
}
export default App;
1 Answer
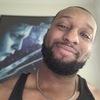
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHi Isaac,
In your App file, you’re passing in ishighScore to your Player component, but in your Player file, you’re trying to access a prop named isHighScore (which does not exist). I think the easiest fix would be to change the name of the prop you’re passing into Player to be isHighScore, but all you really have to do is make sure all the names sync up.
Try that and see if it helps.
If that doesn’t fix it, post a link to your repo so that I can run your app.
Isaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsIsaac Arnold
Front End Web Development Techdegree Graduate 15,863 PointsThanks so much Brandon! Such a simple oversight - how annoying hahaha. Thanks again!
Brandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,660 PointsHaha. I get it. Sometimes all it takes is a fresh set of eyes.