Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial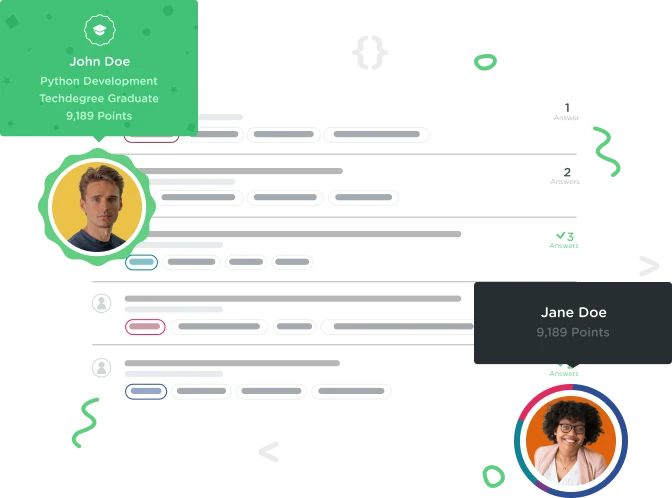

Keenan Smith
Python Development Techdegree Student 5,487 PointsClass method
im pretty sure im doing this completely wrong at this point. Maybe im not reading the instructions right.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, morse):
new_list = []
for i in morse:
if i == "dash":
new_list.append('_')
elif i == "dot":
new_list.append('.')
return new_list
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer
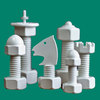
Steven Parker
231,248 PointsYou're pretty close, but I see two issues:
If you iterate over the argument directly, the loop will extract each character individually. So you probably want to split the string into a list of words first.
Then instead of returning the new pattern itself, the instructions ask you to return a new class instance with the correct pattern in it.
Keenan Smith
Python Development Techdegree Student 5,487 PointsKeenan Smith
Python Development Techdegree Student 5,487 PointsSo would the class instance I return be cls? Or would it be Letter()? I am confused about having a new class instance created.
Steven Parker
231,248 PointsSteven Parker
231,248 PointsYou can create a new class instance like this: