Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial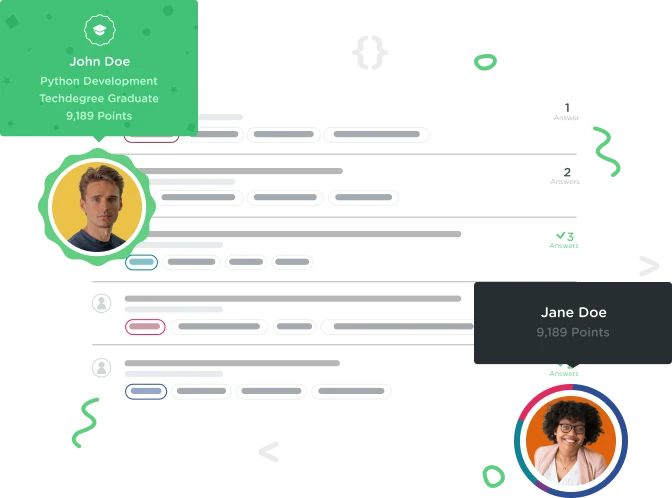

John Reilly
10,800 PointsClass Updating Parent information in a Child Class
Hello All,
I'm messing about with Classes in Python and was wanting to know how do I update information which has been initialized in Parent class in a Child Class.
I'm basically trying to create a standard Space Marine template (Parent Class) with generic stats. Then creating Chapters (Child Class) which are will update these generic stats.
class Space_Marine():
def __init__(self, name, WS = 30, BS = 30, S = 30, T = 30, AG = 30, INT = 30,
PER = 30, WP = 30, FEL = 30):
self.name = name
self.WS = WS
self.BS = BS
self.S = S
self.T = T
self.AG = AG
self.INT = INT
self.PER = PER
self.WP = WP
self.FEL = FEL
class Space_Wolf(Space_Marine):
def __init__(self,name): # This __init__ is clearly not the right solution.
#Update self.WS = self.WS + 5
#Update self.FEL = self.FEL + 5
Player1 = Space_Marine("John")
Player2 = Space_Wolf("Wolfy")
print(Player1.name)
print(Player2.name + " WS is " + str(Player2.WS))
I have commented in the Space_Wolf class what I would like to do. It appears that when I put init in the child class and run it. It will create only the object with those attributes, which obviously defeats the purpose of inheritance.
Hopefully you all understood this rambling, if not please do say, I'll try to make things clearer if necessary. Also big thanks in advanced :D
"For Russ! For the Wolftime!"
1 Answer
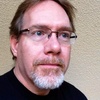
Chris Freeman
Treehouse Moderator 68,423 PointsThe first step is to call the parent __init__()
from the child __init__()
. This can be done with super()
:
Space_Wolf.__init__()
would look something like this:
def __init__(self, *args, **kwargs):
super(Space_Wolf, self).__init__(*args, **kwargs)
# local customization of values here
self.WS += 5
self.FEL += 5
"*args
" handles name
and "**kwargs
" handles the rest