Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial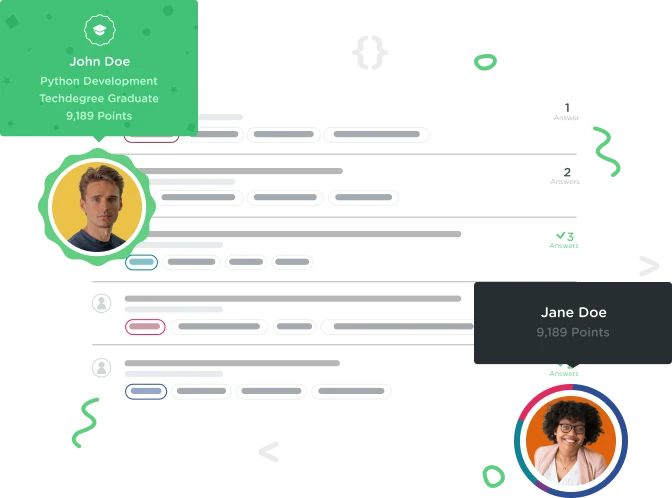

rcyn12
365 PointsClass vs Object
I understand that class is like a blueprint that can make different objects with different methods (right?), but I don't understand how that would appear in code. In this lesson Integer is a class using the method parseInt to do string-> integer. So given this info, would console be another class with methods learned so far of printf and readLine? Or is console an object in this case? Can classes be used as objects sometimes and vice versa?
1 Answer
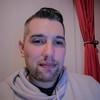
Daniel Hartin
5,311 PointsHi,
You're absolutely right a class is the instructions on how to build an object and object is the result of running that code..... this blew my mind when I first got into coding.
The best example I ever heard was Lego, the mould that makes the lego piece would be the class, this is the code you write which says how the object (piece of Lego) should act and what values the piece should have (state) such as number of bumps and colour etc. The Lego piece on the other hand is the actual object and when you call methods on this object you potentially change or get at the state of that object (there are other things such as static methods which effect the entire class, and other bits and bobs which are pointless explaining right now).
Every single line of code you write in Java is within an object, Java is very strict and unlike some other languages you can't write anything outside of a class so you're using them all the time right now.
To give you some code to explain the Lego scenario above see the below
public class Lego{
private int mNumberOfBumps;
private Colour mColour; //Notice how this is another class!! we can even use classes inside other classes
public Lego(){
//This is a constructor and is the code we run when we say that we want to make a new Lego Piece
mNumberOfBumps = 4;
mColour = Colour.Blue //This is just an example so ignore the fact that there is no Colour class
}
public int getNumberOfBumps(){
//this is a method which we can call on any OBJECT we create from this class to give us some information about the
//state of the object
return mNumberOfBumps;
}
}
//Somewhere else in our code we can write
Lego legoPiece = new Lego(); //This runs the construtor function which is a special function and creates a new Lego //object which we assign to a variable called legoPiece.
//This object can then run the methods of the class as needed
int howManyBumpsDoYouHave = legoPiece.getNumberOfBumps();
I hope this helps in what is a fairly hard concept to grasp, once it clicks though you'll see this makes programming so much easier and allows you to write smaller chunks of code to make even the largest problem attainable with smaller chunks breaking down the problem so you can visualise it easy.
Daniel
Justin Cantley
18,068 PointsJustin Cantley
18,068 PointsI believe you are referring to Java and not Javascript. You should post this question in the Java forum. I think you'll find the answer you're looking for there.