Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial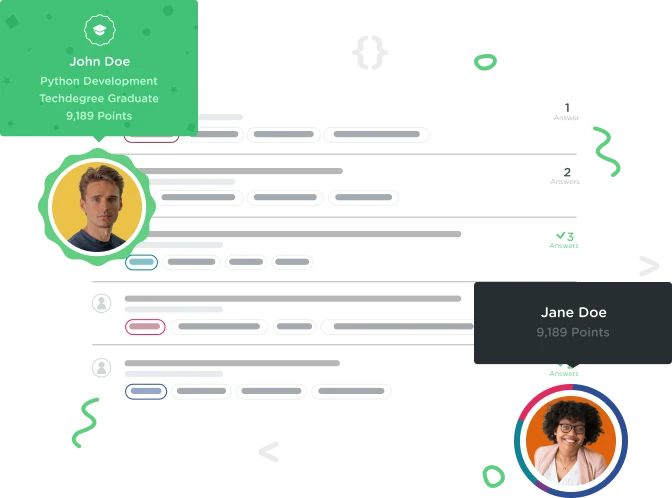
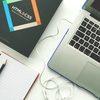
Bekzod Rakhmatov
7,419 PointsClass vs Struct
Hi there! I am a bit confused using class and structure. Does anyone know that why when I use struct and declare some member values into it and assigning it to new constant variable Xcode is automatically showing the member values inside the struct but when I tried in class Xcode is not showing its inside members so why is it happening anybody faced this question before?
EX: struct User { var fullName: String var email: String var age: Int } var someUser = User(fullName: "Bekzod", email: "s", age: 21)
The above code for structure
class User { var fullName: String var email: String var age: Int } var someUser = User(fullName: "Bekzod", email: "s", age: 21)
This one is for class :) Thanks for responding!
1 Answer

Jon Brantingham
7,860 PointsThe main difference between classes and structs are that structs are value types and so values are copied over, while classes are reference types. Classes also have inheritance, so you can have subclasses that have the same methods and variables as their superclass.
When you assign the value of one struct to another, it actually copies the data. In the code below, you'll see MyStruct has a variable "a". I assign this to firstStruct and give it the value "Stuff", and then copy it to secondStruct. I then change the firstStruct.a to "New Stuff", but secondStruct.a stays as "Stuff".
This is not the same as the class. Notice when I do the same thing, when I change firstClass.a to "New Stuff", secondClass.a changes as well.
As far as the class, you need to explicitly state what the init method is, unless you only have optionals.
Try copying the code below into a playground to see how it works.
struct MyStruct {
var a: String
}
var firstStruct = MyStruct(a: "Stuff")
var secondStruct = firstStruct
secondStruct.a
// "Stuff"
firstStruct.a = "New Stuff"
secondStruct.a
// "Stuff"
class MyClass {
var a: String
init(a: String) {
self.a = a
}
}
var firstClass = MyClass(a: "Stuff")
var secondClass = firstClass
secondClass.a
// "Stuff"
firstClass.a = "New Stuff"
secondClass.a
// "New Stuff"
Bekzod Rakhmatov
7,419 PointsBekzod Rakhmatov
7,419 PointsThanks
Bekzod Rakhmatov
7,419 PointsBekzod Rakhmatov
7,419 PointsSo do we need to use init method in class all the time?
Caleb Kleveter
Treehouse Moderator 37,862 PointsCaleb Kleveter
Treehouse Moderator 37,862 PointsIf you don't have a preset value for all your class's properties, then yes, you have to use an init. Structs automatically create the init for you.