Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial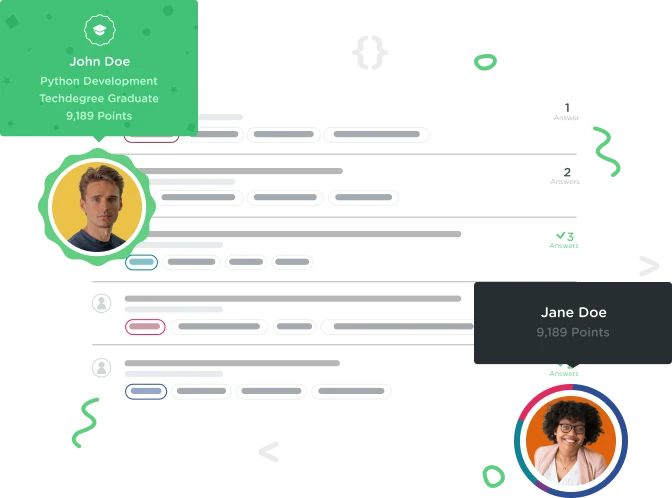

tytyty
4,974 Pointsclasses and methods challenge
OMG Even though I am comfortable with class methods I spent hours trying to figure out the calculation in the method LOL This wording threw me for a loop hehe "(Within the method add points to the width and height properties)." I couldn't figure out if you meant the word "points" or to actually ADD pixel points in a designator init after the class object.
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) {
width = (width + points) // hated this!!
height = (height + points) // hated this!!
}
}
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
}
3 Answers
William Li
Courses Plus Student 26,868 PointsYou're on the right track. Here's the correction you should make to your incrementBy
method.
func incrementBy(points: Double) {
self.width += points
self.height += points
}
In your original code
width = (width + points) // hated this!!
height = (height + points) // hated this!!
incrementBy
method has no idea what width
or height
is, because when you write them like that, the method will assume that they're function parameters you pass in, but since the only parameter you pass in is points
, incrementBy
method doesn't know what to do and where width
or height
come from, thus give you compile-time error. Use self.width
, self.height
to refer to the width and height property of the object and incrementBy
won't be complaining.
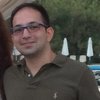
Mohsen Lotfi
2,694 Pointsdoing what William suggested but i,m getting compiler error
Binary operator '+=' cannot be applied to two Double operands
class Button {
let width: Double
let height: Double
init(width: Double, height:Double) {
self.width = width
self.height = height
}
func incrementBy(points: Double) {
self.width += width + points
self.height += height + points
}
}

tytyty
4,974 PointsMohsen, you are adding an extra width and height.
func incrementBy(points: Double) {
self.width += points // remove the word width
self.height += points / remove the word height
}
tytyty
4,974 Pointstytyty
4,974 Pointsoh thanks! We haven't been taught that syntax on the iOS track I'm on. I mean using that operator within an init method.
In my challenge with my code I got a success message so I think it was just looking for the basic structure like I had and then I guess in later lessons we'll be doing what you showed me.
Thanks Will