Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial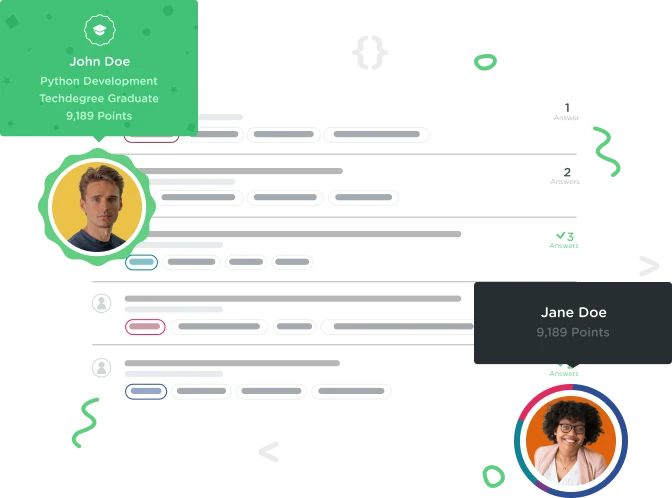

Mahvish Irfan
449 PointsClasses, constructors, instantiating objects and methods. What are they?
Lots of complicated programming words. Can someone please break it down easily for me?
8 Answers
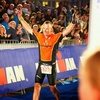
Steve Hunter
57,712 Points"A constructor is the method that you will run when you instantiate the class before the object is returned to the caller. Again, instantiation happens when you create the new instance of the class using the new
keyword. This is a good place to stick information about the initial setup of the object."
Is it that that's confusing you?
OK. So, we have a class which is a blueprint for creating instances of that class. Doing this is called instantiation, it is also referred to as 'creating an object', 'making an instance' etc. There's lots of terminology that can be used. Let's worry about what an object or instance is. An object or instance is a unique version of the class, it is an example of what the class creates, as a blueprint or template. It is the real thing rather than the template itself.
Example required .. erm ... let's say you've got a machine that stamps metal into plates. So, the shape of that stamp is like the class. It isn't a plate but it knows how to create one. The object/instance is an actual plate that gets created when the stamp presses the sheet metal. There can be many, many individual plates produced from this one stamp and they can be stamped out of different thicknesses of metal; that depends what sheet metal you put against the template, but they are all, basically, made the same way. OK - that's one example lamely overdone.
We now have some idea what an instance is and that means we know that instantiate is the verb used to describe creating an instance. So, what's this constructor? Like Craig said, it is a method. That's like a bit of code that does something rather than a bit of code that stores something. A method produces something. A constructor is a specific bit of code that every class has and what it does is build the instance/object. Let's get back to plates ... like that example isn't old already!
In our plate factory, the constructor is the process that gets some metal, puts it in the right place then moves the stamp to press out a plate-shaped object. Craig mentioned that "This is a good place to stick information about the initial setup of the object". So, our constructor could select what type of metal to use (I dunno ... copper, tin, steel, whatever!) and its thickness, perhaps (thin, thick, something else(?!)), then create a plate by applying the template to the metal The end result is a plate that matches the "initial setup of the object" delivered to whoever requested it to be made. Or, as Craig put it, "returned to the caller".
Let's make this even less clear by trying to create some dummy code for our dodgy plate-making example ...
public class Plate{
private int thickness;
private Metal madeOf;
// This is the constructor:
public Plate(int howThick, Metal useMetal){
thickness = howThick;
madeOf = useMetal;
}
}
Plate myPlate = new Plate(2, copper)
(Yes, I made up a Metal class - forgive me!)
This is probably pointless as Craig's course will do a far better job of explaining all this, but it's worth a shot. Here we've replicated the lame plate example in (probably incorrect) Java code - it isn't supposed to be perfect! The person wanting the plate, "the caller", can pass details of what type of plate he wants, "initial setup", into the constructor which will create a plate-shaped object, "instantiate", and give that to the user, "return to the caller".
We can see how, in code, we can create unique objects with differing properties but by using the same template. We could add more properties; color, diameter, shape etc. I didn't as I've done the example to death already. But the key concepts here are the stamp itself - it makes plates, as many plates as you want - and the constructor which allows you to tailor the properties of your plate(s).
We've covered the class, methods, the constructor, instances & objects, instantiation, the caller and we've learned I know nothing about the manufacture of plates, or any other form of crockery, for that matter. But I hope that has shed a little light on the part of the video that was causing you a problem. If not, that's my fault; sorry! Honestly, just crack on with the courses, you will get the hang of all this in no time.
Have a great day!
Steve.
cc. Craig Dennis - I may have confused one of your students ... sorry!
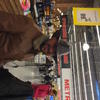
Isam Al-Abbasi
2,058 PointsSteve even though you were so thorough with your answer and you did great job but I had to say that I confused me even more and I had to stop reading because my brain can't seems to handle all this new information :D sorry!!! I'm super new to programming and I begin to think that this is not for me :( I was so eager to learn but it's just too much information to keep up!!
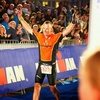
Steve Hunter
57,712 PointsHi Isam,
Sorry to have confused you. Which bits are causing the problem? There's a lot of esoteric concepts involved here, so let's break it down to the points that are confusing you.
Steve.
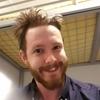
Chris Tvedt
3,795 PointsNever give up! If this is something you want to learn, you can! Go for it! Never stop trying and asking questions!
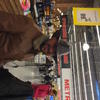
Isam Al-Abbasi
2,058 PointsHi Steve, I really appreciate your concerns for helping me out to understand Java basics but no matter how many times I watch videos about objects, classes and contractors I still don't seem to get the hang of it!! maybe because I am not a native speaker... I don't know!! I mean I still don't know how to type the correct code to build classes or objects even though I did watched objects basics video!! I think I need more time to digest the information slowly and keep reading more about it.
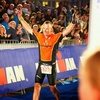
Steve Hunter
57,712 PointsI think repetition is key; repeating the concepts by progressing through the courses will make the seemingly complex issues feel more familiar. Stick at it, Isam, you will get there, I am sure.

Mahvish Irfan
449 PointsAll,
Thank you so much for your help. I have taken a pause from coding at the moment but really feel thankful for your assistance! Your time and effort is really appreciated.
Mahvish
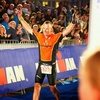
Steve Hunter
57,712 Points
No problem.
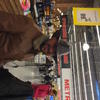
Isam Al-Abbasi
2,058 PointsThank you so much Steve... I really appreciate your help and concerns... I will do the best I can and hopefully one day I will get all the pieces together and get more familiar with this language :)
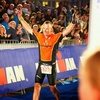
Steve Hunter
57,712 PointsHi Isam,
I know everyone learns differently, so feel free to completely ignore me! (Most people do!! ) I found that covering the different concepts like class, objects etc across different languages helped. So, working through some Java courses, then doing a bit of Android (which is great fun!) and then, maybe, doing some of the Swift basics courses helped me. I think that's because you get the same concept explained to you by a different teacher in a slightly different way.
It helped me - it might be worth trying. Failing that; ask in the Community pages. There's lots of good people out here willing to give their time and explain concepts in their own way. That will certainly help. Remember, you can tag people with an @ symbol in the forum if want a specific person to see your post.
I hope that helps a little.
Steve.
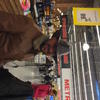
Isam Al-Abbasi
2,058 PointsI will try to do everything you suggested Steve except ignoring you part :) I think I will do the opposite and keep tagging you with my future questions :D So you suggest that I take a break from Java courses now and check out other courses like Android and Swift? do they share the same concepts? I'm afraid that I will loose what I have learnt about Java so far!!
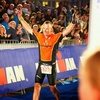
Steve Hunter
57,712 PointsYou won't lose the concepts at all - Android is based within the Java framework in the first place, plus they're all object oriented languages, so you'll meet classes, instances, constructors, objects etc. etc. just in a different variant. These languages are very similar at the base level - they all deal with data types like strings, integers, dates etc. They just implement solutions handling the concepts slightly differently - the concepts are the same. Consider trying that at some point - perhaps not now. And, feel free to tag me in your questions.

Sarah Kavanagh
791 PointsSteve I just wanted to say thank you so much for your example. My head was in pain the last few hours trying to comprehend "what the hell is this stupid constructor doing and why is it in my life?" I was getting quite angry about it.....until I saw your answer. Again thank you so much, I understand now. I can now go to bed in peace and move on with my life.
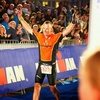
Steve Hunter
57,712 PointsNo problem, Sarah. I'm glad it helped!
Steve.
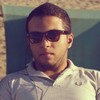
Noor Elharty
2,630 Pointsthanks steve , your explanation helped me a lot , i was really having hard times getting these concepts , i think it begins to make sense now , still feeling a little overwhelmed though but i hope it gets better as i move forward in course ... thanks again ..
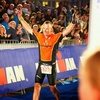
Steve Hunter
57,712 PointsKeep at it, Noor - you'll be fine!
Mahvish Irfan
449 PointsMahvish Irfan
449 PointsSteve Hunter okay, I think I get it. I have to re-read this a bunch of times until it sticks. Thanks so, so much for your thorough response. Very much appreciated.
Jonathan Nott
538 PointsJonathan Nott
538 PointsThis definitely helped, thank you!!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem.