Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial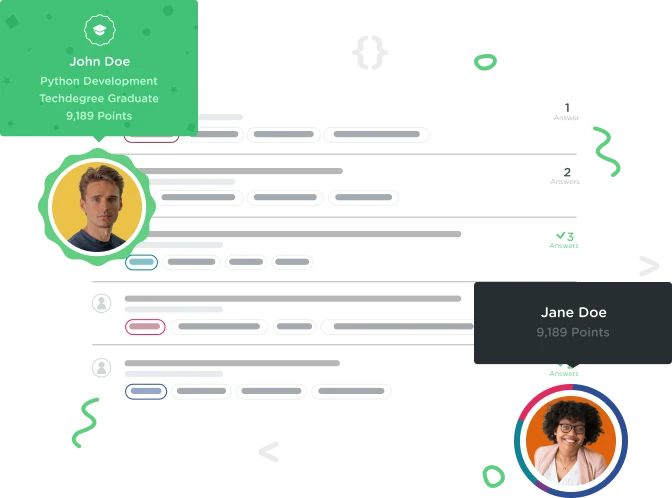

Fred Lawrence
3,833 PointsClasses with Custom Types
I don't know where I am going wrong. Help. Please.
In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude as values.
For this task we want to create a class named Business. The class contains two stored properties: name of type String and location of type Location.
In the initialiser method pass in a name and an instance of Location to set up the instance of Business. Using the initialiser, create an instance and assign it to a constant named Business.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init() {
self.location = Location()
self.name = "HK"
}
let someBusiness = Business()
3 Answers
Rodrigo Chousal
16,009 PointsHey Fred,
The init method you wrote is missing arguments. You should have arguments for your init method so you can assign these arguments to the stored properties of your class. Next, when initialising the constant someBusiness
, you should input some values to create the instance of Business
.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
let someBusiness = Business(name: "HK", location: Location(latitude: 144.5, longitude: 13.4))
Hope this helps, Rodrigo

Fred Lawrence
3,833 PointsVery grateful for your help, thanks.

Anthia Tillbury
3,388 PointsI have the following, and while it will compile in Swift, it will not work on Treehouse's web compiler:
struct Location {
let latitude: Double
let longitude: Double
class Business {
let name: String
let location: Location
init(name: String, latitude: Double, longitude: Double) {
self.name = name
self.location = Location(latitude: latitude, longitude: longitude)
}
}
let someBusiness = Business(name: "simon", latitude: 22.2, longitude: 12.0)
}
Anyway, I'm unsure where I am going wrong.

Seth Roope
6,471 PointsYou probably figured this out by now, but the "let someBusiness" declaration should be outside of the "Location" struct.
Cheers