Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial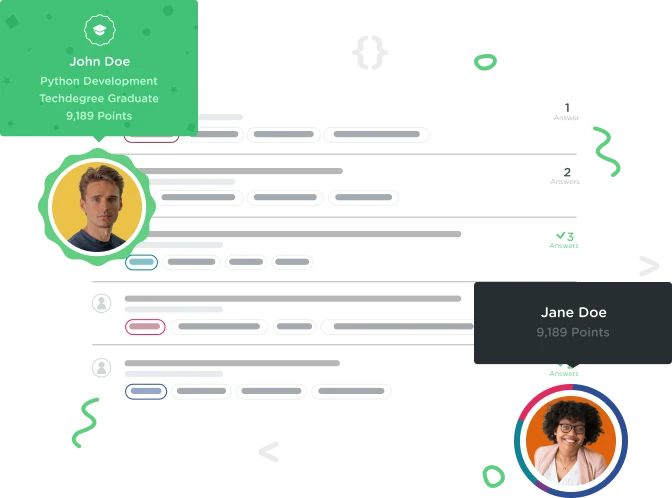
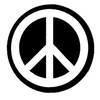
john larson
16,594 PointsclassList.contains vs .contains
These two lines look like they would do the same thing to me
listItem.classList.contains("editMode")
listItem.contains(".editMode")
I'm going through this lesson line by line, looking up every thing . Based on what I found these look like they would accomplish the same thing. But I can't be sure if I'm missing something.
2 Answers
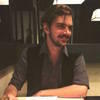
Marco Amadio
4,882 PointsIf you use a class, like ".editMode", as parameter for contains method, it should throw this error:
Uncaught TypeError: Failed to execute 'contains' on 'Node': parameter 1 is not of type 'Node'.
That's because contains method only accepts nodes as parameter. If you want to use contains method on a node your code should be similar to:
var elements = document.getElementsByClassName('editMode');
for ( i in elements ) {
if ( listItem.contains(elements[i]) ) {
label.innerText = editInput.value;
} else {
editInput.value = label.innerText;
}
}
Otherwise, if you use classList, the code you posted should work fine.
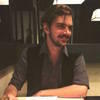
Marco Amadio
4,882 PointsHi John.
In this case
element.classList.contains(class)
"contains" is a "classList"'s method. "classList" returns class name/s of an element, "contains" method returns a boolean value that tell if the element contains the specified class name.
Instead, in this other case
node1.contains(node2)
"contains" method returns a boolean value that indicate if a node is a descendant of a specified node. In the previous example, "contains" method evaluates if node2 is a descendant of node1.
Hope it helps. Cheers!
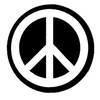
john larson
16,594 Pointsin the example I found for contains: element.contains('.class') was used. So wouldn't the element containing .class be a descendant of element? Which as I'm seeing it is the same as element.classList.contains('class') ? I'm just thinking in this instance, not really beyond that. This is how it will be used...
var containsClass = listItem.classList.contains("editMode");
// alt to the above line
// var containsClass = listItem.contains(".editMode");
if(containsClass){
label.innerText = editInput.value;
} else{
editInput.value = label.innerText;
}
wouldn't the conditional still work?