Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial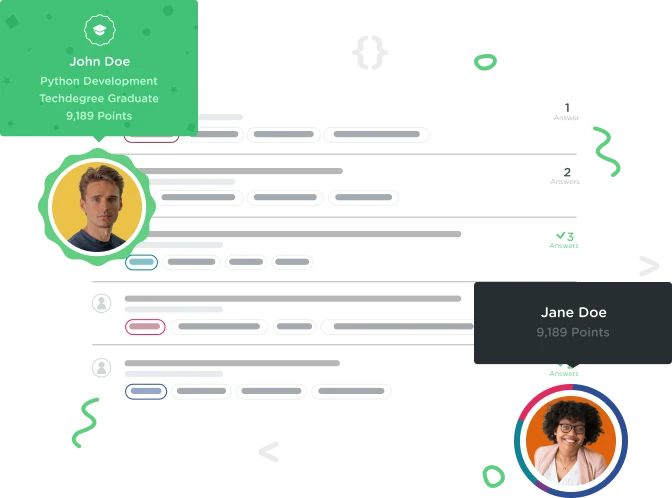
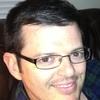
Brian Hudson
14,785 Points'classmethod' object not callable
In the "Construction Zone" challenge, I copied and pasted all of the code from the challenge (including my solution, which passed) into my console. When I tried to test the from_string method I got an error message.
Here is how I attempted to test the from_string method:
ns = from_string(Letter, 'dash-dot-dash-dash-dot')
And here is the error I received:
TypeError: 'classmethod' object is not callable
So, the classmethod is defined, and so is the parent class (Letter). Why is this classmethod error popping up? Am I not calling the from_string method correctly?
5 Answers

Rich Zimmerman
24,063 PointsWithout seeing the entire code to be sure. If you're calling a class' method, you need to define which class is you're calling it from. Something like:
Class Foo:
self.bar():
return baz
# You'd can't just call bar() alone. You'd have to define which class' method is it like:
a = Foo.bar()
Does this help?
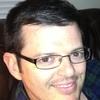
Brian Hudson
14,785 PointsThanks for the reply Rich. I'm trying to call test a method inside a class method. Here's the code I copied and pasted into Terminal:
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, string):
convert_list = string.split('-')
output = []
for i in convert_list:
if i == 'dash':
output.append('_')
elif i == 'dot':
output.append('.')
return cls(output)
And here's the method I'm trying to test:
ns = from_string(Letter, 'dot-dash-dot-dot-dash-dot')
When I type "ns" at the command prompt, I'm expecting to see output of ['.', '', '.', '.', '', '.']. Instead I'm getting the TypeError.
TypeError: 'classmethod' object is not callable
So, again, just not sure what I'm doing wrong.

Rich Zimmerman
24,063 PointsIt should work with
ns = Letter.from_string('dot-dash-dot-dot-dash-dot')
I believe
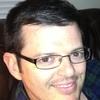
Brian Hudson
14,785 PointsThat one gave me
AttributeError: type object 'Letter' has no attribute 'from_string'

Rich Zimmerman
24,063 PointsSorry, I was doing some googling here.. I'm not entirely sure.
I did come across one stack overflow question with the same issue where the person had an error in his module import lines at the top of the file.
Are you importing the Letter class from another module? If so, how?

jdee
6,655 PointsBrian, I am looking through comments on this challenge to find an answer to your question. I had a similar one in that i initially tried calling the super class' (Letter) from_string() method from an S() instance. I couldn't get it to work. Then, I found an answer I posted here: https://teamtreehouse.com/community/debugging-3
The answer calls it without S(), i.e., only using Letter(). So, I went with that for now, but was confused as to the purpose of including the S class at all in that challenge....probably to confuse dummies like me ;)
Lastly, I, too, did lots of research trying to figure out how, but I never found a solution with class methods using @classmethod
:(