Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial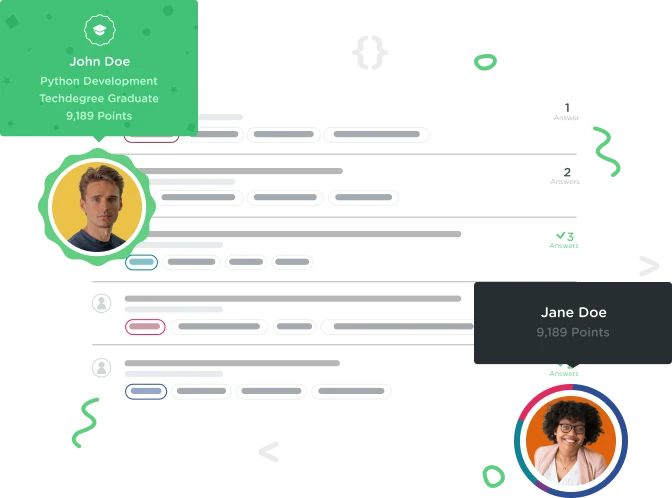

leonardo valdes
12,384 Points@classmethod output question
I passed this code challenge but I was still confused on how classmethods work. I tried to use the from_string method using the string passed, but when I print it it prints the original string and not the dots and dashes that I was expecting. Am I using the class method incorrectly? What should I be doing?
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, string):
entry = string.split('-')
pattern = []
for blip in entry:
if blip == 'dot':
pattern.append('.')
else:
pattern.append('_')
return cls(pattern)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
test = Letter.from_string('dash-dot-dash-dot')
print(test)
1 Answer

andren
28,558 PointsWhen you pass something that is not a string into the print
function it will automatically converting it to a string by calling the str
method on the object. So when you call print(test)
you are actually printing out whatever the test
object's __str__
method produces.
The __str__
method on the Letter
class loops through the pattern and returns the decoded version of it. That is why you get that version printed out.
leonardo valdes
12,384 Pointsleonardo valdes
12,384 PointsI figured out that using test.pattern outputs what I was looking for. So, that brings up another question: why does it print the original string when I put print(test), but not when i put print(test.pattern)? What is the difference?