Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial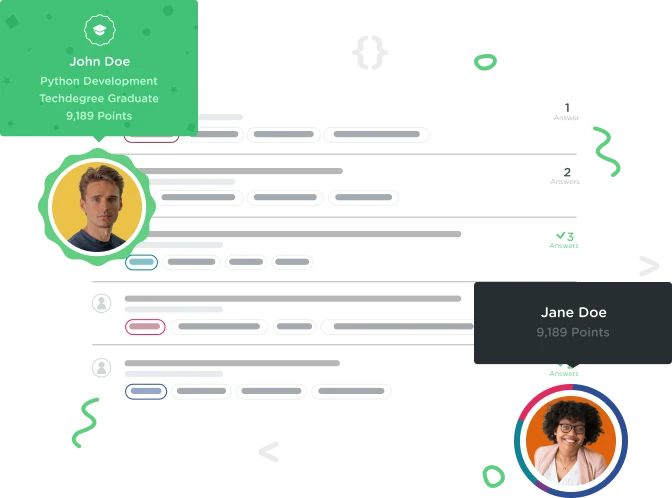

Amy Tomey
12,847 Pointsclassroom size doesn't change...
why isn't the second log saying 2?
Here is my console log:
stevenJ is in the classroom
sarah is in the classroom
stevenS is in the classroom
classroom size: 3
classroom size: 3
Here is the code:
'use strict';
let classroom = new Set();
let stevenJ = { name: 'Steven', age: 22 },
sarah = { name: 'Sarah', age: 23 },
stevenS = { name: 'Steven', age: 22 };
classroom.add(stevenJ);
classroom.add(sarah);
classroom.add(stevenS);
classroom.add(sarah);
if (classroom.has(stevenJ)) console.log('stevenJ is in the classroom');
if (classroom.has(sarah)) console.log('sarah is in the classroom');
if (classroom.has(stevenS)) console.log('stevenS is in the classroom');
console.log('classroom size:', classroom.size);
classroom.delete('stevenJ');
console.log('classroom size:', classroom.size);
1 Answer
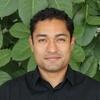
Juan Luna Ramirez
9,038 PointsYou are trying to delete the string 'stevenJ'
instead of the object { name: Steven, age: 22 }
which is saved to the stevenJ
variable. Nothing is being deleted since there is no 'stevenJ'
string in your Set.
'use strict';
let classroom = new Set();
let stevenJ = { name: 'Steven', age: 22 },
sarah = { name: 'Sarah', age: 23 },
stevenS = { name: 'Steven', age: 22 };
classroom.add(stevenJ);
classroom.add(sarah);
classroom.add(stevenS);
classroom.add(sarah);
if (classroom.has(stevenJ)) console.log('stevenJ is in the classroom');
if (classroom.has(sarah)) console.log('sarah is in the classroom');
if (classroom.has(stevenS)) console.log('stevenS is in the classroom');
console.log('classroom size:', classroom.size);
// remove the quotes so that you are passing in the reference to the stevenJ object
console.log(classroom.delete(stevenJ));
console.log('classroom size:', classroom.size);